用c语言写一个贪吃蛇
时间: 2023-11-16 16:06:15 浏览: 37
好的,下面是一个简单的贪吃蛇游戏的C语言代码。代码中使用了Windows.h库和conio.h库,所以只能在Windows系统下运行。
```
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <Windows.h>
#define WIDTH 50 // 游戏画面宽度
#define HEIGHT 20 // 游戏画面高度
int score = 0; // 得分
int speed = 150; // 初始速度,值越小越快
// 蛇的结构体
struct Snake {
int x;
int y;
struct Snake *next;
};
struct Snake *head = NULL; // 蛇的头结点
struct Snake *tail = NULL; // 蛇的尾结点
// 食物的结构体
struct Food {
int x;
int y;
};
struct Food food;
// 初始化游戏
void init() {
// 初始化蛇
head = (struct Snake*)malloc(sizeof(struct Snake));
head->x = WIDTH / 2;
head->y = HEIGHT / 2;
head->next = NULL;
tail = head;
// 初始化食物
srand((unsigned)time(NULL));
food.x = rand() % (WIDTH - 2) + 1;
food.y = rand() % (HEIGHT - 2) + 1;
// 清空屏幕
system("cls");
}
// 显示画面
void display() {
// 清空屏幕
system("cls");
// 显示蛇
struct Snake *p = head;
while (p != NULL) {
printf("O");
p = p->next;
}
printf("\n");
// 显示边框和食物
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (i == 0 || i == HEIGHT - 1 || j == 0 || j == WIDTH - 1) {
printf("#");
}
else if (i == food.y && j == food.x) {
printf("*");
}
else {
printf(" ");
}
}
printf("\n");
}
// 显示得分
printf("Score: %d\n", score);
}
// 蛇移动
void move(char direction) {
// 记录蛇尾的位置
int preX = tail->x;
int preY = tail->y;
// 移动蛇头
switch (direction) {
case 'w':
head->y--;
break;
case 's':
head->y++;
break;
case 'a':
head->x--;
break;
case 'd':
head->x++;
break;
}
// 判断是否吃到食物
if (head->x == food.x && head->y == food.y) {
// 新增一个蛇节点
struct Snake *newNode = (struct Snake*)malloc(sizeof(struct Snake));
newNode->x = preX;
newNode->y = preY;
newNode->next = NULL;
tail->next = newNode;
tail = newNode;
// 重新生成食物
srand((unsigned)time(NULL));
food.x = rand() % (WIDTH - 2) + 1;
food.y = rand() % (HEIGHT - 2) + 1;
// 增加得分,并加速
score++;
if (speed > 50) {
speed -= 5;
}
}
else {
// 移动蛇尾
struct Snake *p = head;
while (p->next != tail) {
p = p->next;
}
p->next = NULL;
tail->x = head->x;
tail->y = head->y;
tail->next = NULL;
head = tail;
tail = p;
}
}
// 判断是否游戏结束
int isGameOver() {
// 判断是否撞到边界
if (head->x == 0 || head->x == WIDTH - 1 || head->y == 0 || head->y == HEIGHT - 1) {
return 1;
}
// 判断是否撞到自己
struct Snake *p = head->next;
while (p != NULL) {
if (head->x == p->x && head->y == p->y) {
return 1;
}
p = p->next;
}
return 0;
}
int main() {
init();
while (1) {
display();
if (_kbhit()) {
char c = _getch();
if (c == 'w' || c == 's' || c == 'a' || c == 'd') {
move(c);
}
}
else {
move(' ');
}
if (isGameOver()) {
printf("Game Over!\n");
system("pause");
break;
}
Sleep(speed);
}
return 0;
}
```
这只是一个简单的贪吃蛇游戏,仅供参考。如果需要更完整的游戏,可以在此基础上进行改进。
相关推荐
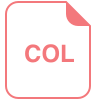
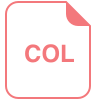
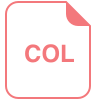
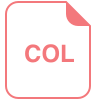
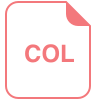









