Python的安装及编译,创建一个语料库,计算文本词汇表,并统计单词出现的次数,过滤文本。并按文体(两个以上)计数词汇,绘制其中情态动词的条件频率分布图和分布表。要求使用文本编辑器创建程序,使用关键字表示函数输出,以python文件命名,编写相应程序。
时间: 2023-09-21 19:06:21 浏览: 44
1. Python的安装及编译
首先,需要在官网下载Python的安装包,根据操作系统选择合适的版本进行安装。
安装完成后,可以在终端或命令行中输入`python`,如果出现Python的版本号,则表示安装成功。
2. 创建一个语料库
语料库指的是用于自然语言处理的文本数据集合。可以使用Python内置的`open`函数来打开一个文本文件并读取其中的内容。
```python
with open('corpus.txt', 'r', encoding='utf-8') as f:
corpus = f.read()
```
上述代码将打开名为`corpus.txt`的文本文件,并将其中的内容读取到`corpus`变量中。
3. 计算文本词汇表,并统计单词出现的次数
可以使用Python中的`collections`模块来统计词频。
```python
import collections
# 分词
words = corpus.split()
# 统计词频
word_freq = collections.Counter(words)
# 打印词频最高的前10个单词
print(word_freq.most_common(10))
```
上述代码将对语料库中的文本进行分词,并使用`collections`模块中的`Counter`函数统计每个单词出现的次数。最后,打印出现频率最高的前10个单词。
4. 过滤文本
在自然语言处理中,常常需要对文本进行过滤,例如去除停用词等。可以使用Python中的`nltk`模块来进行过滤。
```python
import nltk
from nltk.corpus import stopwords
# 下载停用词
nltk.download('stopwords')
# 过滤停用词
filtered_words = [word for word in words if word.lower() not in stopwords.words('english')]
# 统计词频
filtered_word_freq = collections.Counter(filtered_words)
# 打印词频最高的前10个单词
print(filtered_word_freq.most_common(10))
```
上述代码将使用`nltk`模块中的`stopwords`函数下载英文停用词,并将文本中的停用词过滤掉。最后,统计过滤后的词频并打印出现频率最高的前10个单词。
5. 按文体(两个以上)计数词汇
可以使用Python中的`nltk`模块中的`corpus`函数来读取不同文体的语料库,并分别对其进行统计。
```python
import nltk
from nltk.corpus import gutenberg, brown
# 读取Gutenberg语料库
gutenberg_words = gutenberg.words()
gutenberg_word_freq = collections.Counter(gutenberg_words)
# 读取Brown语料库
brown_words = brown.words()
brown_word_freq = collections.Counter(brown_words)
# 打印Gutenberg语料库中出现频率最高的前10个单词
print(gutenberg_word_freq.most_common(10))
# 打印Brown语料库中出现频率最高的前10个单词
print(brown_word_freq.most_common(10))
```
上述代码将分别读取Gutenberg语料库和Brown语料库,并统计每个文体中单词的出现频率。最后,打印出现频率最高的前10个单词。
6. 绘制情态动词的条件频率分布图和分布表
可以使用Python中的`nltk`模块中的`ConditionalFreqDist`函数来计算情态动词的条件频率分布,并使用`matplotlib`模块来绘制图表。
```python
import nltk
from nltk.corpus import brown
import matplotlib.pyplot as plt
# 计算情态动词的条件频率分布
cfd = nltk.ConditionalFreqDist((genre, word) for genre in brown.categories() for word in brown.words(categories=genre) if word in ['can', 'could', 'may', 'might', 'must', 'will'])
# 绘制条件频率分布图
cfd.plot()
# 绘制条件频率分布表
cfd.tabulate()
```
上述代码将使用`nltk`模块中的`ConditionalFreqDist`函数计算情态动词在不同文体中的条件频率分布,并使用`matplotlib`模块绘制图表。最后,使用`tabulate`函数打印条件频率分布表。
相关推荐
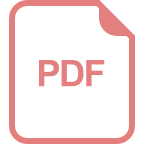
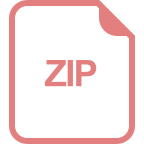














