python查找图片中“外置SD卡分区”文字的位置并把位置打印出来
时间: 2024-09-26 20:17:04 浏览: 35
在Python中,你可以使用OCR(Optical Character Recognition,光学字符识别)技术结合图像处理库如PIL(Pillow)、 pytesseract 和 OpenCV 来定位图片中特定的文字,比如“外置SD卡分区”。Tesseract是一个开源的OCR引擎,可以用于读取文字。
以下是一个简单的示例步骤:
1. 首先,你需要安装必要的库:
```bash
pip install Pillow pytesseract opencv-python-headless
```
2. 然后,你可以使用以下代码来定位文字:
```python
import cv2
from PIL import Image
import pytesseract
# 打开图片文件
img = cv2.imread('your_image_path.jpg')
# 将BGR图像转换为灰度图像,因为tesseract更适合处理灰度图像
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 对图像进行二值化,提高 OCR 效率
thresh = cv2.threshold(gray_img, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
# 使用pytesseract进行OCR识别,并获取文字
text = pytesseract.image_to_string(Image.fromarray(thresh))
# 查找特定文字 "外置SD卡分区"
target_text = "外置SD卡分区"
position = text.find(target_text)
if position != -1:
print(f"'{target_text}' 的位置是:{position}")
else:
print("目标文字未找到")
# 如果需要精确位置,你还可以使用 pytesseract.get_boxes() 获取每个识别区域的矩形坐标,然后进一步分析
```
注意,这个过程可能对图像质量和OCR引擎的训练数据敏感,对于复杂或低质量的图片,结果可能会不准确。
阅读全文
相关推荐
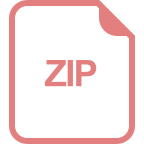
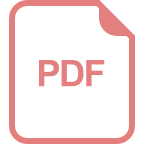
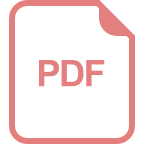









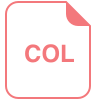
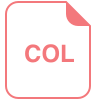
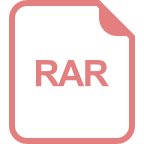
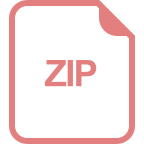
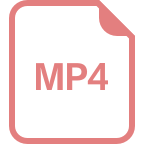