写一个java创意小程序,要求包含GUI知识
时间: 2024-01-25 16:02:48 浏览: 25
好的,我可以给您提供一个简单的Java GUI程序,它可以随机生成一个颜色,并在窗口上显示该颜色的名称。
首先,我们需要创建一个 JFrame 对象,用于显示我们的GUI。然后,我们需要创建一个 JPanel 对象,将其添加到 JFrame 中,以容纳我们的GUI元素。接下来,我们可以将一个 JLabel 添加到 JPanel 中,以显示随机生成的颜色的名称。
下面是完整的代码:
```
import java.awt.Color;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
public class ColorGeneratorGUI {
private static final String[] COLORS = { "红色", "橙色", "黄色", "绿色", "蓝色", "紫色" };
public static void main(String[] args) {
JFrame frame = new JFrame("颜色生成器");
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel = new JPanel();
frame.add(panel);
JLabel colorLabel = new JLabel("点击下面的按钮生成一个随机颜色");
panel.add(colorLabel);
JButton button = new JButton("生成颜色");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
Random random = new Random();
int index = random.nextInt(COLORS.length);
String colorName = COLORS[index];
Color color = getColor(colorName);
panel.setBackground(color);
colorLabel.setText(colorName);
}
});
panel.add(button);
frame.setVisible(true);
}
private static Color getColor(String colorName) {
switch (colorName) {
case "红色":
return Color.RED;
case "橙色":
return Color.ORANGE;
case "黄色":
return Color.YELLOW;
case "绿色":
return Color.GREEN;
case "蓝色":
return Color.BLUE;
case "紫色":
return Color.MAGENTA;
default:
return Color.BLACK;
}
}
}
```
运行该程序,您应该可以看到一个窗口,其中包含一个显示“点击下面的按钮生成一个随机颜色”的标签和一个生成颜色的按钮。单击按钮后,窗口的背景颜色将变为随机生成的颜色,并且标签将显示该颜色的名称。
希望这个小程序可以帮助您学习Java GUI编程!
相关推荐
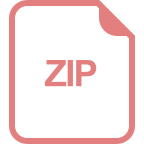
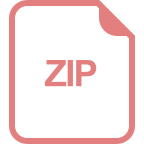






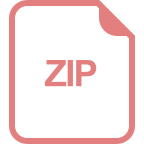
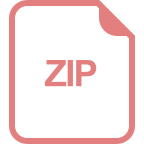
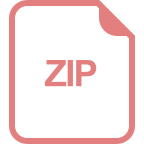