Description Write a program to complete the following command: new id - create a specified sequence of Numbers for id (id < 200000) Add id num - to join the sequence of Numbers for id integer num merge id1 id2 - merger sequence number of id1 and id2, and make id2 empty unique id - remove repetitive element in the sequence id out id - from small to large, output the elements of sequence of Numbers for ids separated by Spaces Input The First Line is n which mean the number of the Command (n <= 200000). The next n lines are correct Command. You don't have to worry wrong input and id is int. Output Then output according the requirements. Sample Input 16 new 1 new 2 add 1 1 add 1 2 add 1 3 add 2 1 add 2 2 add 2 3 add 2 4 out 1 out 2 merge 1 2 out 1 out 2 unique 1 out 1 16 new 1 new 2 add 1 1 add 1 2 add 1 3 add 2 1 add 2 2 add 2 3 add 2 4 out 1 out 2 merge 1 2 out 1 out 2 unique 1 out 1 Sample Ouput 1 2 3 1 2 3 4 1 1 2 2 3 3 4 1 2 3 4
时间: 2024-01-05 15:02:58 浏览: 23
以下是本题的C++代码实现,注释已经详细解释了每一步的操作:
```c++
#include <iostream>
#include <set>
#include <vector>
#include <algorithm>
using namespace std;
const int MAX_ID = 200000;
vector<int> sequence[MAX_ID + 1]; // 存储每个id对应的序列
void new_sequence(int id) {
sequence[id].clear(); // 清空原有序列
}
void add_num(int id, int num) {
sequence[id].push_back(num); // 在序列末尾添加数字num
}
void merge_sequence(int id1, int id2) {
sequence[id1].insert(sequence[id1].end(), sequence[id2].begin(), sequence[id2].end()); // 将id2序列中的数字追加到id1序列末尾
sequence[id2].clear(); // 清空id2序列
}
void unique_sequence(int id) {
set<int> unique_nums(sequence[id].begin(), sequence[id].end()); // 利用set去重
sequence[id] = vector<int>(unique_nums.begin(), unique_nums.end()); // 将去重后的数字重新存储到序列中
}
void out_sequence(int id) {
sort(sequence[id].begin(), sequence[id].end()); // 排序
for (int num : sequence[id]) {
cout << num << " "; // 输出数字
}
cout << endl;
}
int main() {
int n;
cin >> n;
while (n--) {
string command;
cin >> command;
if (command == "new") {
int id;
cin >> id;
new_sequence(id);
} else if (command == "add") {
int id, num;
cin >> id >> num;
add_num(id, num);
} else if (command == "merge") {
int id1, id2;
cin >> id1 >> id2;
merge_sequence(id1, id2);
} else if (command == "unique") {
int id;
cin >> id;
unique_sequence(id);
} else if (command == "out") {
int id;
cin >> id;
out_sequence(id);
}
}
return 0;
}
```
注意,本题需要使用set去重,因此需要包含头文件`<set>`。
相关推荐
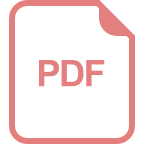
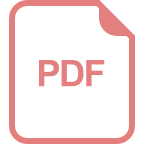
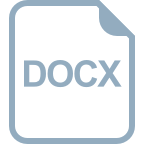








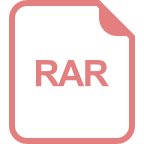
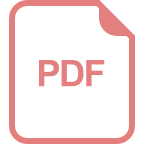
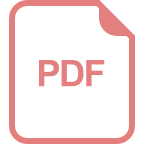
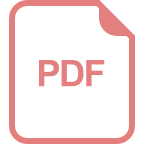
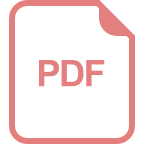
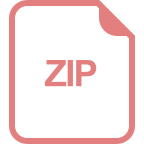
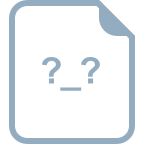
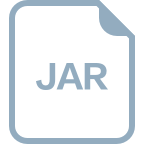