编写一个程序,用于描述啮齿动物信息。其中Rodent(抽象类)、老鼠类(Mouse)和松鼠类(Squirrel)之间的UML图如下所示: image.png 注:测试类必须命名为Main并声明为public,其他类不能声明为public,否则程序编译报错。 测试类提示代码:
时间: 2024-10-29 15:13:22 浏览: 39
根据您提供的UML图,我们可以创建一个包含三个类的程序结构:抽象的`Rodent`类作为父类,具象的`Mouse`和`Squirrel`类作为子类。以下是相关的Java代码:
```java
// 抽象类 Rodent
abstract class Rodent {
protected String name;
protected int age;
public Rodent(String name, int age) {
this.name = name;
this.age = age;
}
// 定义抽象方法 eat()
abstract void eat();
// 共享的 getter 和 setter 方法
public String getName() { return name; }
public int getAge() { return age; }
}
// 子类 Mouse
class Mouse extends Rodent {
private double tailLength;
public Mouse(String name, int age, double tailLength) {
super(name, age);
this.tailLength = tailLength;
}
@Override
void eat() {
System.out.println("老鼠正在吃食物...");
}
public double getTailLength() { return tailLength; }
}
// 子类 Squirrel
class Squirrel extends Rodent {
private String furColor;
public Squirrel(String name, int age, String furColor) {
super(name, age);
this.furColor = furColor;
}
@Override
void eat() {
System.out.println("松鼠正在吃坚果...");
}
public String getFurColor() { return furColor; }
}
// 测试类 Main
public class Main {
public static void main(String[] args) {
// 创建鼠标和松鼠对象,并展示它们的信息
Rodent mouse = new Mouse("米奇", 2, 7.5);
Rodent squirrel = new Squirrel("索尼克", 3, "灰色");
displayInfo(mouse);
displayInfo(squirrel);
// 测试 eat() 方法
mouse.eat();
squirrel.eat();
}
// 非公开的静态方法,仅用于演示
public static void displayInfo(Rodent rodent) {
System.out.println("啮齿动物信息:");
System.out.println("名字: " + rodent.getName());
System.out.println("年龄: " + rodent.getAge());
if (rodent instanceof Mouse) {
Mouse mouse = (Mouse) rodent;
System.out.println("尾巴长度: " + mouse.getTailLength());
} else if (rodent instanceof Squirrel) {
Squirrel squirrel = (Squirrel) rodent;
System.out.println("毛色: " + squirrel.getFurColor());
}
System.out.println("----------");
}
}
```
在这个程序中,`Main` 类负责创建和测试`Rodent`、`Mouse`和`Squirrel`的实例,而`displayInfo()`方法用于显示这些动物的具体信息。
阅读全文
相关推荐
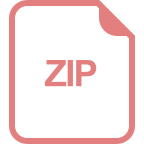
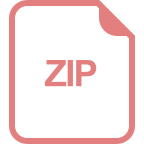
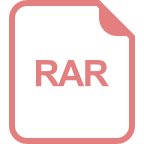
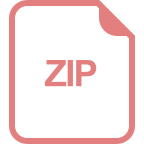
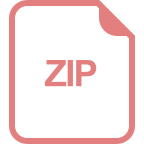
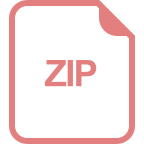
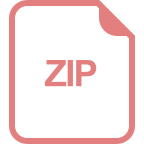









