绘制烟花粒子的
时间: 2023-09-10 10:13:32 浏览: 79
绘制烟花粒子可以使用canvas或者WebGL进行实现。
以下是使用canvas绘制烟花粒子的示例代码:
```html
<canvas id="fireworks"></canvas>
```
```javascript
// 获取canvas元素和上下文
const canvas = document.getElementById('fireworks');
const ctx = canvas.getContext('2d');
// 设置canvas尺寸
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
// 烟花粒子类
class FireworkParticle {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.color = color;
this.radius = 2;
this.opacity = 1;
this.velocity = {
x: Math.random() * 4 - 2,
y: Math.random() * 4 - 2
};
}
// 更新烟花粒子属性
update() {
this.x += this.velocity.x;
this.y += this.velocity.y;
this.opacity -= 0.01;
this.radius += 0.01;
}
// 绘制烟花粒子
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.radius, 0, Math.PI * 2, false);
ctx.fillStyle = `rgba(${this.color}, ${this.opacity})`;
ctx.fill();
}
// 判断烟花粒子是否消失
isDead() {
return this.opacity <= 0;
}
}
// 烟花类
class Firework {
constructor(x, y, color) {
this.x = x;
this.y = y;
this.color = color;
this.particles = [];
this.lifeSpan = 50;
}
// 更新烟花属性
update() {
if (this.lifeSpan > 0) {
this.lifeSpan--;
} else {
// 创建烟花粒子
for (let i = 0; i < 50; i++) {
const particle = new FireworkParticle(this.x, this.y, this.color);
this.particles.push(particle);
}
}
// 更新烟花粒子属性
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].update();
if (this.particles[i].isDead()) {
this.particles.splice(i, 1);
}
}
}
// 绘制烟花
draw() {
for (let i = 0; i < this.particles.length; i++) {
this.particles[i].draw();
}
}
}
const fireworks = [];
// 创建烟花
function createFirework(x, y) {
const color = `${Math.floor(Math.random() * 255)},${Math.floor(Math.random() * 255)},${Math.floor(Math.random() * 255)}`;
const firework = new Firework(x, y, color);
fireworks.push(firework);
}
// 绘制烟花
function drawFireworks() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
for (let i = 0; i < fireworks.length; i++) {
fireworks[i].update();
fireworks[i].draw();
}
}
// 点击canvas创建烟花
canvas.addEventListener('click', (event) => {
createFirework(event.clientX, event.clientY);
});
// 定时绘制烟花
setInterval(drawFireworks, 10);
```
该示例中,通过FireworkParticle类和Firework类分别实现了烟花粒子和烟花的属性和方法,使用createFirework函数和canvas的click事件来创建烟花,使用setInterval函数定时绘制烟花效果。
阅读全文
相关推荐
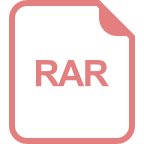

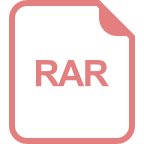
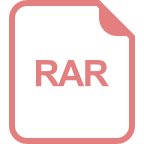
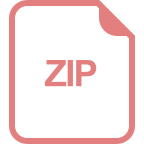
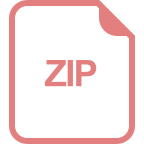
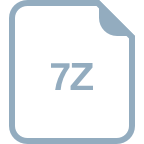
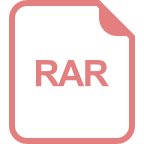
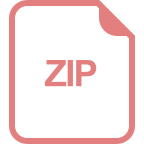
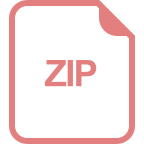
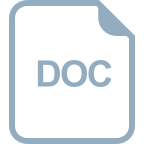
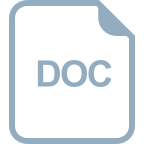
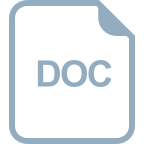


