python GridSearchCV调参
时间: 2023-10-28 17:52:03 浏览: 99
的步骤是什么?
为使用 GridSearchCV 进行调参,你需要完成以下步骤:
1. 导入所需模块和数据。
2. 定义模型和模型参数空间。
3. 定义评分函数(即模型调参时的指标)。
4. 确定交叉验证策略。
5. 创建 GridSearchCV 对象,并对模型进行训练和调参。
6. 访问最佳参数和最佳模型,并进行预测和评估。
这些步骤中的每一个都需要注意一些细节,例如模型的参数需要怎样设置,交叉验证应如何进行等等。因此,在进行模型调参时,需要学习和掌握这些知识,以便更好地应用 GridSearchCV。
相关问题
python GridSearchCV调参例子
好的,关于 Python 中 GridSearchCV 的调参例子,你可以参考以下代码:
```python
from sklearn.model_selection import GridSearchCV
from sklearn.ensemble import RandomForestClassifier
from sklearn.datasets import make_classification
# 生成数据
X, y = make_classification(n_samples=1000, n_features=4,
n_informative=2, n_redundant=0,
random_state=0, shuffle=False)
# 定义模型
rf = RandomForestClassifier(random_state=0)
# 定义要进行调参的参数范围
param_grid = {'max_depth': [2, 3, 5, 10],
'min_samples_split': [2, 3, 5],
'n_estimators': [50, 100, 200]}
# 定义 GridSearchCV 对象,并进行训练
grid_search = GridSearchCV(estimator=rf, param_grid=param_grid, cv=5)
grid_search.fit(X, y)
# 打印最优参数和最优得分
print("Best parameters found: ", grid_search.best_params_)
print("Best score found: ", grid_search.best_score_)
```
这是一个简单的随机森林分类器的例子,它使用 GridSearchCV 对分类器进行调参。在这个例子中,我们使用了 `max_depth`、`min_samples_split` 和 `n_estimators` 三个参数,并定义了它们的取值范围。GridSearchCV 将在这个参数范围内搜索最佳的参数组合,并返回最佳得分和最佳参数组合。
希望这个例子能够帮助到你,如果有其他问题,可以继续问我。
python 实现SkLearn中基于GridSearchCV调参的MLPRegressor算法
下面是一个使用GridSearchCV调参的MLPRegressor算法的Python实现:
```python
from sklearn.neural_network import MLPRegressor
from sklearn.model_selection import GridSearchCV
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
import pandas as pd
# 读取数据
data = pd.read_csv('data.csv')
# 划分数据集和测试集
X_train, X_test, y_train, y_test = train_test_split(data.iloc[:, :-1], data.iloc[:, -1], test_size=0.2, random_state=42)
# 设置参数范围
param_grid = {'hidden_layer_sizes': [(50, 50, 50), (50, 100, 50), (100,)],
'activation': ['tanh', 'relu'],
'solver': ['sgd', 'adam'],
'alpha': [0.0001, 0.05],
'learning_rate': ['constant', 'adaptive']}
# 构建模型
mlp = MLPRegressor(random_state=42)
# 使用GridSearchCV进行参数调优
grid_search = GridSearchCV(mlp, param_grid=param_grid, cv=5, scoring='neg_mean_squared_error')
grid_search.fit(X_train, y_train)
# 输出最优参数
print("Best parameters:{}".format(grid_search.best_params_))
# 使用最优参数构建模型
mlp = MLPRegressor(hidden_layer_sizes=grid_search.best_params_['hidden_layer_sizes'],
activation=grid_search.best_params_['activation'],
solver=grid_search.best_params_['solver'],
alpha=grid_search.best_params_['alpha'],
learning_rate=grid_search.best_params_['learning_rate'],
random_state=42)
# 训练模型
mlp.fit(X_train, y_train)
# 预测模型
y_pred = mlp.predict(X_test)
# 输出结果
print("RMSE: {}".format(np.sqrt(mean_squared_error(y_test, y_pred))))
```
在上述代码中,首先我们读取了数据并将其划分为训练集和测试集。然后,我们设置了需要调优的参数范围,并且使用GridSearchCV进行参数调优。在调优过程中,我们使用了5折交叉验证,并使用均方误差作为评估指标。最后,我们使用最优参数构建模型,并训练和预测模型。最终,输出了均方根误差作为评估结果。
需要注意的是,这里的数据集需要自行准备。同时,该代码只是一个示例,实际使用时可能需要根据数据集的情况进行适当的调整。
阅读全文
相关推荐
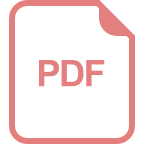
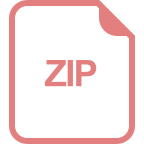
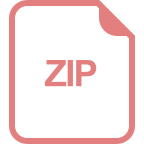

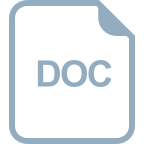








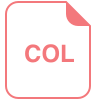


