结合“计算机组成原理”课程加法器设思想,用8086汇编语言十六位乘法指令完成三十二位 无符号数乘法(结果64位),数据存储在内存中,由自定义数据段指定地址。在此基础之上, 进一步讨论32位带符号数的乘法设计。
时间: 2023-12-31 20:04:31 浏览: 40
对于三十二位无符号数乘法,可以使用8086汇编语言的MUL指令。该指令将一个无符号的八位或十六位数与AL或AX中的内容相乘,并将结果存储在AX或DX:AX中。因此,可以使用两个MUL指令来完成三十二位无符号数的乘法。
假设数据存储在内存中的地址为0x1000,高位在前,低位在后。下面是一个示例程序:
```
assume cs:code, ds:data
data segment
num1 dw 0x1234 ; 低位在前,高位在后
num2 dw 0x5678 ; 低位在前,高位在后
result dw 0, 0, 0, 0 ; 结果64位,低位在前,高位在后
data ends
code segment
start:
mov ax, data
mov ds, ax ; 设置数据段寄存器
; 低16位相乘
mov ax, word ptr [num1]
mul word ptr [num2]
mov word ptr [result], ax ; 存储低16位
; 高16位相乘
mov ax, word ptr [num1 + 2]
mul word ptr [num2]
add word ptr [result + 2], ax ; 存储次高16位
adc word ptr [result + 4], dx ; 存储最高16位
; 显示结果
mov ah, 9
lea dx, [result]
int 21h
mov ah, 4ch
int 21h
code ends
```
在上面的程序中,首先定义了三个变量:`num1`和`num2`表示要相乘的两个数,`result`表示结果。注意,`result`变量是一个四字(即64位)的数组,低位存储在`result[0]`和`result[1]`中,高位存储在`result[2]`和`result[3]`中。这里使用了`result dw 0, 0, 0, 0`来初始化`result`变量,将其全部置为0。
在代码段中,首先设置数据段寄存器,然后使用`MUL`指令相乘。对于低16位的相乘,直接将`num1`和`num2`中的数加载到AX寄存器中,然后执行`MUL`指令即可。结果存储在AX寄存器中,将其存储到`result[0]`和`result[1]`中即可。
对于高16位的相乘,先将`num1`的高16位加载到AX寄存器中,然后执行`MUL`指令,将结果存储在DX:AX中。此时,DX中存储的就是次高16位的结果,将其加到`result[2]`中即可。由于乘法可能会产生进位,因此需要使用`ADC`指令将进位加到`result[4]`中。
最后,使用`INT 21h`中断显示结果,然后结束程序。
对于32位带符号数的乘法,可以使用乘法展开法。具体来说,将两个带符号数分别表示为两个32位无符号数`num1_high:num1_low`和`num2_high:num2_low`,然后使用上面的方法计算四个无符号数的乘积,即:
```
a = num1_high * num2_high
b = num1_high * num2_low
c = num1_low * num2_high
d = num1_low * num2_low
```
最后将它们相加即可得到结果:
```
result = (a << 32) + (b + c) << 16 + d
```
注意,在上述计算中,需要处理四个数的符号位,以及相加时可能出现的进位。具体实现可以参考下面的示例程序:
```
assume cs:code, ds:data
data segment
num1_high dd 0x12345678
num1_low dd 0x9abcdef0
num2_high dd 0x56789abc
num2_low dd 0xdef01234
result dq 0, 0 ; 结果64位,低位在前,高位在后
data ends
code segment
start:
mov ax, data
mov ds, ax ; 设置数据段寄存器
; 计算a
mov eax, dword ptr [num1_high]
imul dword ptr [num2_high]
mov dword ptr [result], eax
mov dword ptr [result + 4], edx
; 计算b和c
mov eax, dword ptr [num1_high]
mul dword ptr [num2_low]
mov dword ptr [result + 8], eax
mov dword ptr [result + 12], edx
mov eax, dword ptr [num1_low]
mul dword ptr [num2_high]
add dword ptr [result + 8], eax
adc dword ptr [result + 12], edx
; 计算d
mov eax, dword ptr [num1_low]
mul dword ptr [num2_low]
add dword ptr [result + 16], eax
adc dword ptr [result + 20], edx
; 处理符号位和进位
mov eax, dword ptr [result + 8]
add eax, dword ptr [result + 12]
adc dword ptr [result + 16], 0
adc dword ptr [result + 20], 0
mov eax, dword ptr [result]
add eax, dword ptr [result + 4]
adc dword ptr [result + 8], 0
adc dword ptr [result + 12], 0
; 显示结果
mov ah, 9
lea dx, [result]
int 21h
mov ah, 4ch
int 21h
code ends
```
在上面的程序中,首先定义了四个带符号数`num1_high:num1_low`和`num2_high:num2_low`,以及一个结果变量`result`。注意,结果变量是一个八字(即64位)的数组,低位存储在`result[0]`和`result[1]`中,高位存储在`result[2]`和`result[3]`中。这里使用了`result dq 0, 0`来初始化`result`变量,将其全部置为0。
在代码段中,首先设置数据段寄存器,然后分别计算四个无符号数的乘积。对于每个乘积,使用`IMUL`或`MUL`指令计算,并将结果存储在`result`数组中。注意,在计算b和c时,需要将结果加到`result[2]`和`result[3]`中,并处理进位。在计算d时,需要将结果加到`result[4]`和`result[5]`中,并处理进位。
最后,将四个无符号数的乘积相加,并处理符号位和进位。使用`INT 21h`中断显示结果,然后结束程序。
相关推荐
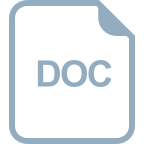
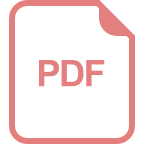
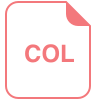
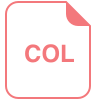
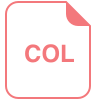











