用pytorch编写基于resnet50的图像能见度检测程序
时间: 2023-06-13 10:05:42 浏览: 65
下面是一个基于ResNet50的图像能见度检测程序,使用PyTorch实现:
```python
import torch
import torch.nn as nn
import torchvision.models as models
class VisibilityDetector(nn.Module):
def __init__(self):
super(VisibilityDetector, self).__init__()
self.resnet = models.resnet50(pretrained=True)
self.fc1 = nn.Linear(1000, 512)
self.fc2 = nn.Linear(512, 1)
def forward(self, x):
x = self.resnet(x)
x = self.fc1(x)
x = nn.ReLU()(x)
x = self.fc2(x)
return x
model = VisibilityDetector()
```
在这个例子中,我们使用了预训练的ResNet50模型作为我们的特征提取器,然后将提取的特征输入到两个全连接层中,最终输出一个标量,表示输入图像的能见度。你可以根据需要修改模型结构和参数。
相关问题
在pytorch基于resnet50的图像能见度检测的代码
以下是基于PyTorch实现的图像能见度检测代码,使用的是ResNet50模型:
```
import torch
import torch.nn as nn
import torchvision.models as models
class VisibilityNet(nn.Module):
def __init__(self):
super(VisibilityNet, self).__init__()
self.resnet = models.resnet50(pretrained=True)
self.fc1 = nn.Linear(1000, 256)
self.relu = nn.ReLU()
self.fc2 = nn.Linear(256, 1)
self.sigmoid = nn.Sigmoid()
def forward(self, x):
x = self.resnet(x)
x = self.fc1(x)
x = self.relu(x)
x = self.fc2(x)
x = self.sigmoid(x)
return x
```
在训练数据集上训练模型时,可以使用以下代码:
```
import torch.optim as optim
import torch.utils.data as data
from torchvision import transforms
# Define transforms for data augmentation
transform = transforms.Compose([
transforms.Resize((224, 224)),
transforms.RandomHorizontalFlip(),
transforms.ToTensor(),
transforms.Normalize((0.485, 0.456, 0.406), (0.229, 0.224, 0.225))
])
# Load the dataset using DataLoader
train_set = data.Dataset(train_data_path, transform=transform)
train_loader = data.DataLoader(train_set, batch_size=batch_size, shuffle=True)
# Initialize the model and optimizer
model = VisibilityNet()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
# Train the model on the training set
for epoch in range(num_epochs):
for i, (images, labels) in enumerate(train_loader):
images = images.to(device)
labels = labels.to(device)
optimizer.zero_grad()
outputs = model(images)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
```
其中,train_data_path是训练数据集的路径,batch_size是每个batch的大小,learning_rate是学习率,num_epochs是训练的轮数。在每个batch训练完后,通过计算损失和反向传播优化模型参数。
在pytorch用resnet50完成对图像进行能见度检测的代码
以下是使用 PyTorch 和 ResNet50 进行图像能见度检测的示例代码:
```python
import torch
import torchvision.models as models
import torchvision.transforms as transforms
from PIL import Image
# 加载ResNet50模型
model = models.resnet50(pretrained=True)
# 设置模型为评估模式
model.eval()
# 图像转换
transform = transforms.Compose([
transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406],
std=[0.229, 0.224, 0.225])
])
# 加载图像
img = Image.open("test_image.jpg")
# 应用变换
img_t = transform(img)
batch_t = torch.unsqueeze(img_t, 0)
# 推理模型
out = model(batch_t)
# 获取预测结果
_, index = torch.max(out, 1)
# 输出结果
if index[0] == 0:
print("能见度较差")
else:
print("能见度良好")
```
在这个例子中,我们首先加载 ResNet50 模型,并将其设置为评估模式。然后,我们使用 PyTorch 的 transforms 模块将图像转换为 PyTorch 张量,并应用一系列变换来归一化图像。
接下来,我们将处理后的图像传递给模型进行推理,并使用 PyTorch 的 max 函数获取预测结果。最后,根据预测结果输出能见度的标签。
请注意,这只是一个简单的示例,实际情况下,您可能需要对代码进行更多的修改来适应您的特定任务和数据集。
相关推荐
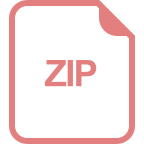
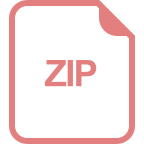
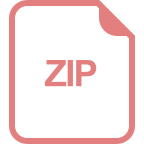
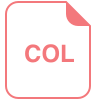

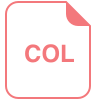









