如何向fstream中写入字符串
时间: 2024-04-29 21:24:05 浏览: 68
可以使用流插入运算符(<<)向fstream中写入字符串。例如:
```cpp
#include <fstream>
#include <iostream>
using namespace std;
int main() {
ofstream outfile("example.txt");
if (outfile.is_open()) {
outfile << "Hello, world!";
outfile.close();
} else {
cout << "Unable to open file";
}
return 0;
}
```
在上面的例子中,我们创建了一个名为example.txt的文件,并使用ofstream类打开了它。然后,我们使用流插入运算符<<向文件中写入了字符串"Hello, world!"。最后,我们关闭了文件。
相关问题
fstream可以写入字符串变量的内容吗
是的,fstream可以写入字符串变量的内容。可以使用fstream对象的`<<`运算符来将字符串写入文件中。例如:
```c++
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main() {
string message = "Hello, World!";
ofstream file("example.txt");
if (file.is_open()) {
file << message;
file.close();
cout << "File written successfully." << endl;
} else {
cout << "Unable to open file." << endl;
}
return 0;
}
```
在这个示例中,我们创建了一个字符串变量`message`,然后使用`ofstream`对象`file`打开了一个名为`example.txt`的文件。我们使用`<<`运算符将`message`写入文件中,然后关闭文件。
fstream可以写入字符串变量的内容,在qt中具体如何实现
在Qt中使用`QFile`和`QTextStream`可以实现文件的读写操作,其中`QTextStream`可以方便地读写字符串变量的内容。
例如,如果要将一个字符串写入文件,可以使用以下代码:
```
QString str = "Hello World!";
QFile file("test.txt");
if (file.open(QIODevice::WriteOnly | QIODevice::Text)) {
QTextStream out(&file);
out << str;
file.close();
}
```
其中,`QIODevice::WriteOnly`表示以只写模式打开文件,`QIODevice::Text`表示以文本格式进行读写。然后创建了一个`QTextStream`对象`out`,并将其绑定到文件对象`file`上,最后使用`<<`运算符将字符串`str`写入文件。
同样地,如果需要从文件中读取字符串,可以使用以下代码:
```
QString str;
QFile file("test.txt");
if (file.open(QIODevice::ReadOnly | QIODevice::Text)) {
QTextStream in(&file);
str = in.readAll();
file.close();
}
```
其中,`QIODevice::ReadOnly`表示以只读模式打开文件,通过`QTextStream`的`readAll()`函数可以将文件中的所有内容读取到字符串变量`str`中。
阅读全文
相关推荐
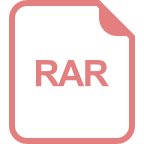
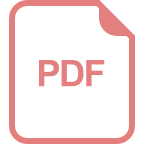
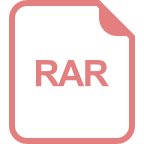













