pyopengl绘制一个带刻度的3维坐标系
时间: 2024-10-27 12:10:41 浏览: 29
在PyOpenGL中绘制一个带刻度的三维坐标系通常涉及到几个步骤:
1. **导入库**:
首先,你需要导入所需的模块,如`OpenGL.GLUT`(用于图形用户界面)、`OpenGL.GL`(处理基本的OpenGL函数)和`numpy`(用于数学计算)。
```python
import OpenGL.GL as GL
import OpenGL.GLUT as GLUT
import numpy as np
```
2. **设置基础结构**:
定义三个轴(X、Y、Z),以及它们的长度和角度。
```python
axis_lengths = (10, 10, 10)
axis_angles = ((1, 0, 0), (0, 1, 0), (0, 0, 1))
```
3. **创建顶点数据**:
创建立方体模型的顶点数据,包括每个轴上的点。
```python
def create_coordinate_axis_vertices():
vertices = []
for axis in axis_lengths:
for i in range(-4, 5):
x = axis * i * np.cos(axis_angles[0])
y = axis * i * np.sin(axis_angles[1])
z = axis * i * np.sin(axis_angles[2])
vertices.append((x, y, z))
return vertices
```
4. **绘制网格**:
使用glBegin()和glEnd()开始和结束顶点数组,然后循环绘制各个面。
```python
def draw_coordinate_axes(vertices):
GL.glLineWidth(2.0) # 设置线条宽度
GL.glColor3f(1, 1, 1) # 白色
GL.glVertexPointer(3, GL.GL_FLOAT, 0, vertices)
GL.glDrawArrays(GL.GL_LINES, 0, len(vertices))
GL.glFlush()
```
5. **添加刻度标记**:
可能需要编写额外的函数来绘制标尺线和数字,这可能涉及更复杂的数学计算和纹理映射技术。
6. **主循环**:
在GLUT的main loop中调用上述函数来更新视图,并显示刻度。
```python
def display():
GL.glClear(GL.GL_COLOR_BUFFER_BIT | GL.GL_DEPTH_BUFFER_BIT)
draw_coordinate_axes(vertices)
# ... 其他绘图操作 ...
GLUT.glutSwapBuffers()
# 然后在main函数中初始化窗口并调用display函数
GLUT.glutInit()
GLUT.glutCreateWindow("3D Coordinate System")
GLUT.glutDisplayFunc(display)
...
```
注意:以上是一个简化的示例,实际应用中可能需要进行一些优化和调整,例如缩放、平移和旋转坐标系等。
阅读全文
相关推荐
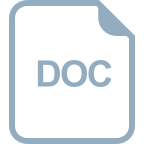
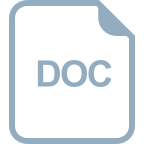
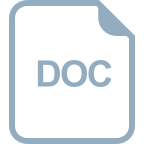
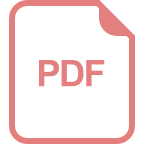



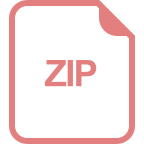
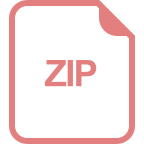
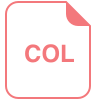
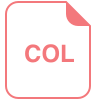
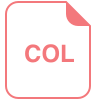
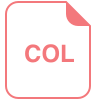
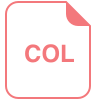
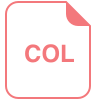
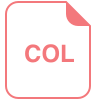
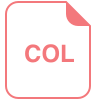
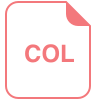
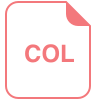