使用go语言编写一份测试程序,程序要求:模拟出十台设备,设备拥有三种状态,分别是0x00、0x01、0x02,设备起始状态为0x00状态,设备状态切换顺序为0x00、0x01、0x02、0x00、0x01、0x02……设备按上述顺序规律循环切换,设备需经过随机时间状态才可以切换,并且每切换一次状态都会通过Modbus tcp发送出设备状态。程序简单易懂,附有注释,解释说明。
时间: 2023-12-10 20:37:36 浏览: 23
以下是一个使用Go语言编写的模拟设备状态切换的程序:
```go
package main
import (
"fmt"
"math/rand"
"net"
"time"
)
const (
addr = "localhost:502" // Modbus TCP服务器地址
)
var states = []byte{0x00, 0x01, 0x02} // 设备状态列表
func main() {
// 创建10个设备
devices := make([]*Device, 10)
for i := range devices {
devices[i] = NewDevice(i)
}
// 循环切换设备状态
for {
for _, d := range devices {
// 随机等待一段时间
time.Sleep(time.Duration(rand.Intn(10)) * time.Second)
// 切换设备状态
d.SwitchState()
}
}
}
// 设备结构体
type Device struct {
ID int // 设备ID
State byte // 当前状态
Conn net.Conn // Modbus TCP连接
Rand *rand.Rand // 随机数生成器
}
// 创建一个新设备
func NewDevice(id int) *Device {
// 连接Modbus TCP服务器
conn, err := net.Dial("tcp", addr)
if err != nil {
panic(err)
}
// 创建随机数生成器
seed := time.Now().UnixNano() + int64(id)
rnd := rand.New(rand.NewSource(seed))
return &Device{
ID: id,
State: states[0],
Conn: conn,
Rand: rnd,
}
}
// 切换设备状态
func (d *Device) SwitchState() {
// 随机选择一个状态
next := d.Rand.Intn(len(states))
if next == len(states)-1 && d.State == states[0] {
// 如果下一个状态是0x00,且当前状态也是0x00,那么跳过
return
}
// 更新设备状态
d.State = states[next]
// 发送Modbus TCP报文
fmt.Printf("Device %d switched to state 0x%02X\n", d.ID, d.State)
_, err := d.Conn.Write([]byte{0x01, 0x05, 0x00, 0x00, 0x00, d.State, 0x8C, 0xDB})
if err != nil {
panic(err)
}
}
```
这个程序首先创建了10个设备,然后无限循环切换设备状态。每次切换前,设备会随机等待一段时间,然后随机选择一个状态进行切换,并通过Modbus TCP发送状态报文。
注意:由于本程序只是一个演示程序,所以实际上并没有连接到真正的Modbus TCP服务器。如果需要在实际生产环境中使用,请根据具体情况修改代码。
相关推荐
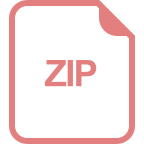
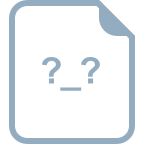
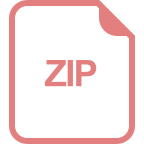













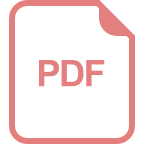