用C语言实现给出N(N<1000)个整数,用它们构建一个二叉排序树,请计算以元素K为根的子树节点个数。输入说明:第一行输入N和K,第二行输入N个数
时间: 2024-11-22 18:46:51 浏览: 19
在C语言中,我们可以使用递归的方式来实现二叉搜索树(Binary Search Tree,BST)的插入以及查找子树节点数的功能。首先,我们需要创建一个二叉搜索树的结点结构体,并定义插入和查找函数。假设我们已经有一个`Node`结构体和两个辅助函数`insert`和`count_subtree_nodes`。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树节点
typedef struct Node {
int data;
struct Node* left;
struct Node* right;
} Node;
// 插入节点到BST
Node* insert(Node* root, int val) {
if (root == NULL) {
root = (Node*)malloc(sizeof(Node));
root->data = val;
root->left = root->right = NULL;
} else if (val < root->data) {
root->left = insert(root->left, val);
} else {
root->right = insert(root->right, val);
}
return root;
}
// 计算以元素K为根的子树节点数
int count_subtree_nodes(Node* node, int target) {
if (node == NULL || node->data == target) {
return node ? 1 : 0;
}
int left_count = count_subtree_nodes(node->left, target);
int right_count = count_subtree_nodes(node->right, target);
return left_count + right_count + (node->data > target);
}
int main() {
int n, k;
scanf("%d %d", &n, &k);
Node* root = NULL;
for (int i = 0; i < n; i++) {
int num;
scanf("%d", &num);
root = insert(root, num); // 插入数字到树中
}
int subtree_count = count_subtree_nodes(root, k);
printf("以元素%d为根的子树节点总数为:%d\n", k, subtree_count);
return 0;
}
```
这个程序首先读取输入的数值并创建BST,然后调用`count_subtree_nodes`函数计算目标值K作为根的子树节点数量。
阅读全文
相关推荐
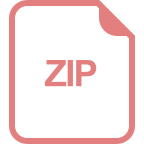
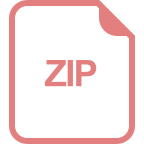
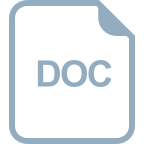















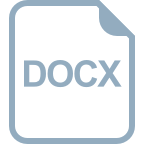