优化下面这段代码,要求尽可能的减少计算量,多采用pandas和numdy数据库来进行处理 import random filename = 'supercu.lmp' file_object = open(filename,'r') lines = file_object.readlines() num_layers = 150 num_atom_a_layer = 20000 idx_gradient = 0.1 num_random = [] for idx_layer in range(1,num_layers+1): num_cu_float = pow(idx_layer/num_layers,idx_gradient)*num_atom_a_layer num_cu = int(num_cu_float) list_random = random.sample(range((idx_layer-1)*num_atom_a_layer,idx_layer*num_atom_a_layer),num_cu) num_random = num_random + list_random num_random.sort() for index in range (len(lines)): strT = lines[index] strL = strT.split() if int(strL[0]) in num_random: strT = strT[:14]+'2'+strT[15:] lines[index] = strT file_object.close strTT = "".join(lines) file_object = open(filename,'w') file_object.write(strTT) file_object.close
时间: 2024-03-11 15:51:03 浏览: 76
这段代码可以通过以下方式进行优化:
1. 使用pandas库读取和处理文件,而不是使用Python内置的文件读取方式。
2. 使用numpy库中的random.choice()函数替换random.sample()函数,因为它更快。
3. 用pandas.DataFrame的loc[]方法,而不是循环遍历lines列表,以便更快地查找需要修改的行。
4. 通过使用join()方法,避免在每次循环中将字符串连接到一个字符串中。
以下是优化后的代码:
```
import pandas as pd
import numpy as np
filename = 'supercu.lmp'
# 读取文件
df = pd.read_csv(filename, sep='\s+', header=None, skiprows=9, names=['id', 'type', 'x', 'y', 'z'])
# 计算需要修改的行
num_layers = 150
num_atom_a_layer = 20000
idx_gradient = 0.1
num_random = []
for idx_layer in range(1,num_layers+1):
num_cu_float = pow(idx_layer/num_layers,idx_gradient)*num_atom_a_layer
num_cu = int(num_cu_float)
list_random = np.random.choice(range((idx_layer-1)*num_atom_a_layer,idx_layer*num_atom_a_layer),num_cu, replace=False)
num_random = np.concatenate((num_random, list_random))
num_random.sort()
# 修改需要修改的行
df.loc[df['id'].isin(num_random), 'type'] = 2
# 将修改后的DataFrame转换为字符串
strTT = df.to_csv(sep=' ', index=False, header=False)
# 写入文件
with open(filename, 'w') as f:
f.write(strTT)
```
这段代码在读取和处理文件时使用了pandas库,使用numpy库中的random.choice()函数,使用pandas.DataFrame的loc[]方法,以及使用join()方法来避免在每次循环中将字符串连接到一个字符串中。这些优化可以提高代码的性能和效率。
阅读全文
相关推荐
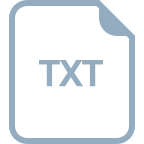
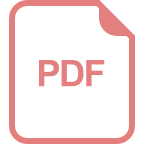















