pthread_arr_t 和 pthread的区别
时间: 2023-08-28 18:19:49 浏览: 75
`pthread_arr_t` 和 `pthread` 都与 POSIX 线程相关,但是它们有不同的含义和用途。
`pthread` 是 POSIX 线程库中的一种数据类型,代表一个线程。`pthread` 类型的变量通常用于创建、启动、管理和同步线程。
`pthread_arr_t` 则是一个自定义的数据类型,它是一个指向 `pthread` 数组的指针。它通常用于在程序中定义一个线程数组,可以用于批量创建和管理多个线程,以及对线程数组进行操作和同步。
因此,`pthread` 和 `pthread_arr_t` 是不同的类型,分别用于表示单个线程和线程数组,它们有不同的用途和操作方式。
相关问题
pthread线程池实现快速排序并计时 c++
好的,这是一个比较复杂的问题,需要分步骤来回答。
首先,我们需要实现一个基本的快速排序算法,可以用以下的代码实现:
```c
void quick_sort(int *arr, int left, int right){
int i, j, pivot, temp;
if(left < right){
i = left;
j = right;
pivot = arr[left];
while(i < j){
while(i < j && arr[j] >= pivot)
j--;
if(i < j)
arr[i++] = arr[j];
while(i < j && arr[i] < pivot)
i++;
if(i < j)
arr[j--] = arr[i];
}
arr[i] = pivot;
quick_sort(arr, left, i - 1);
quick_sort(arr, i + 1, right);
}
}
```
接下来,我们需要实现一个线程池。这里我们使用pthread库实现。以下是线程池的代码:
```c
typedef struct{
int *arr;
int left;
int right;
} Task;
typedef struct{
Task *task_queue;
int queue_head;
int queue_tail;
int queue_size;
pthread_mutex_t queue_lock;
pthread_cond_t queue_not_empty;
pthread_cond_t queue_not_full;
pthread_t *threads;
int thread_count;
int is_shutdown;
} ThreadPool;
void thread_pool_init(ThreadPool *pool, int thread_count, int queue_size){
pool->thread_count = thread_count;
pool->queue_size = queue_size;
pool->queue_head = pool->queue_tail = 0;
pool->is_shutdown = 0;
pool->threads = (pthread_t *)malloc(sizeof(pthread_t) * thread_count);
pool->task_queue = (Task *)malloc(sizeof(Task) * queue_size);
pthread_mutex_init(&(pool->queue_lock), NULL);
pthread_cond_init(&(pool->queue_not_empty), NULL);
pthread_cond_init(&(pool->queue_not_full), NULL);
for(int i = 0; i < thread_count; i++)
pthread_create(&(pool->threads[i]), NULL, thread_func, (void *)pool);
}
void thread_pool_add_task(ThreadPool *pool, Task task){
pthread_mutex_lock(&(pool->queue_lock));
while((pool->queue_tail + 1) % pool->queue_size == pool->queue_head){
pthread_cond_wait(&(pool->queue_not_full), &(pool->queue_lock));
}
pool->task_queue[pool->queue_tail] = task;
pool->queue_tail = (pool->queue_tail + 1) % pool->queue_size;
pthread_cond_signal(&(pool->queue_not_empty));
pthread_mutex_unlock(&(pool->queue_lock));
}
void thread_pool_shutdown(ThreadPool *pool){
pthread_mutex_lock(&(pool->queue_lock));
pool->is_shutdown = 1;
pthread_cond_broadcast(&(pool->queue_not_empty));
pthread_mutex_unlock(&(pool->queue_lock));
for(int i = 0; i < pool->thread_count; i++)
pthread_join(pool->threads[i], NULL);
free(pool->threads);
free(pool->task_queue);
pthread_mutex_destroy(&(pool->queue_lock));
pthread_cond_destroy(&(pool->queue_not_empty));
pthread_cond_destroy(&(pool->queue_not_full));
}
void *thread_func(void *arg){
ThreadPool *pool = (ThreadPool *)arg;
Task task;
while(1){
pthread_mutex_lock(&(pool->queue_lock));
while(pool->queue_head == pool->queue_tail && !pool->is_shutdown){
pthread_cond_wait(&(pool->queue_not_empty), &(pool->queue_lock));
}
if(pool->is_shutdown){
pthread_mutex_unlock(&(pool->queue_lock));
pthread_exit(NULL);
}
task = pool->task_queue[pool->queue_head];
pool->queue_head = (pool->queue_head + 1) % pool->queue_size;
pthread_cond_signal(&(pool->queue_not_full));
pthread_mutex_unlock(&(pool->queue_lock));
quick_sort(task.arr, task.left, task.right);
}
}
```
最后,我们需要实现计时功能。可以用以下代码实现:
```c
#include <sys/time.h>
double get_time(){
struct timeval tv;
gettimeofday(&tv, NULL);
return tv.tv_sec + tv.tv_usec / 1000000.0;
}
```
现在我们需要将这些代码组合起来,实现一个快速排序的线程池,并计时。以下是完整的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <sys/time.h>
typedef struct{
int *arr;
int left;
int right;
} Task;
typedef struct{
Task *task_queue;
int queue_head;
int queue_tail;
int queue_size;
pthread_mutex_t queue_lock;
pthread_cond_t queue_not_empty;
pthread_cond_t queue_not_full;
pthread_t *threads;
int thread_count;
int is_shutdown;
} ThreadPool;
void quick_sort(int *arr, int left, int right){
int i, j, pivot, temp;
if(left < right){
i = left;
j = right;
pivot = arr[left];
while(i < j){
while(i < j && arr[j] >= pivot)
j--;
if(i < j)
arr[i++] = arr[j];
while(i < j && arr[i] < pivot)
i++;
if(i < j)
arr[j--] = arr[i];
}
arr[i] = pivot;
quick_sort(arr, left, i - 1);
quick_sort(arr, i + 1, right);
}
}
void thread_pool_init(ThreadPool *pool, int thread_count, int queue_size){
pool->thread_count = thread_count;
pool->queue_size = queue_size;
pool->queue_head = pool->queue_tail = 0;
pool->is_shutdown = 0;
pool->threads = (pthread_t *)malloc(sizeof(pthread_t) * thread_count);
pool->task_queue = (Task *)malloc(sizeof(Task) * queue_size);
pthread_mutex_init(&(pool->queue_lock), NULL);
pthread_cond_init(&(pool->queue_not_empty), NULL);
pthread_cond_init(&(pool->queue_not_full), NULL);
for(int i = 0; i < thread_count; i++)
pthread_create(&(pool->threads[i]), NULL, thread_func, (void *)pool);
}
void thread_pool_add_task(ThreadPool *pool, Task task){
pthread_mutex_lock(&(pool->queue_lock));
while((pool->queue_tail + 1) % pool->queue_size == pool->queue_head){
pthread_cond_wait(&(pool->queue_not_full), &(pool->queue_lock));
}
pool->task_queue[pool->queue_tail] = task;
pool->queue_tail = (pool->queue_tail + 1) % pool->queue_size;
pthread_cond_signal(&(pool->queue_not_empty));
pthread_mutex_unlock(&(pool->queue_lock));
}
void thread_pool_shutdown(ThreadPool *pool){
pthread_mutex_lock(&(pool->queue_lock));
pool->is_shutdown = 1;
pthread_cond_broadcast(&(pool->queue_not_empty));
pthread_mutex_unlock(&(pool->queue_lock));
for(int i = 0; i < pool->thread_count; i++)
pthread_join(pool->threads[i], NULL);
free(pool->threads);
free(pool->task_queue);
pthread_mutex_destroy(&(pool->queue_lock));
pthread_cond_destroy(&(pool->queue_not_empty));
pthread_cond_destroy(&(pool->queue_not_full));
}
void *thread_func(void *arg){
ThreadPool *pool = (ThreadPool *)arg;
Task task;
while(1){
pthread_mutex_lock(&(pool->queue_lock));
while(pool->queue_head == pool->queue_tail && !pool->is_shutdown){
pthread_cond_wait(&(pool->queue_not_empty), &(pool->queue_lock));
}
if(pool->is_shutdown){
pthread_mutex_unlock(&(pool->queue_lock));
pthread_exit(NULL);
}
task = pool->task_queue[pool->queue_head];
pool->queue_head = (pool->queue_head + 1) % pool->queue_size;
pthread_cond_signal(&(pool->queue_not_full));
pthread_mutex_unlock(&(pool->queue_lock));
quick_sort(task.arr, task.left, task.right);
}
}
double get_time(){
struct timeval tv;
gettimeofday(&tv, NULL);
return tv.tv_sec + tv.tv_usec / 1000000.0;
}
int main(){
int arr[1000000];
for(int i = 0; i < 1000000; i++)
arr[i] = rand() % 1000000;
ThreadPool pool;
thread_pool_init(&pool, 4, 10000);
double start_time = get_time();
Task task;
task.arr = arr;
task.left = 0;
task.right = 999999;
thread_pool_add_task(&pool, task);
thread_pool_shutdown(&pool);
double end_time = get_time();
printf("Time: %lf\n", end_time - start_time);
return 0;
}
```
运行程序,可以看到输出的时间,就是快速排序的时间。注意,线程池的线程数和任务队列大小可以根据自己的需求进行调整。
pthread 线程池实现快速排序并计时 c++
下面是使用pthread线程池实现快速排序并计时的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <sys/time.h>
#define THREAD_NUM 8
#define ARRAY_SIZE 10000000
typedef struct {
int *arr;
int left;
int right;
} quicksort_args_t;
typedef struct {
quicksort_args_t *args;
pthread_t thread;
} worker_t;
typedef struct {
worker_t *workers;
int worker_num;
pthread_mutex_t mutex;
pthread_cond_t cond;
int task_count;
int task_index;
int done;
} thread_pool_t;
void init_thread_pool(thread_pool_t *pool, int worker_num);
void submit_task(thread_pool_t *pool, quicksort_args_t *args);
void join_thread_pool(thread_pool_t *pool);
void *worker(void *args);
void quicksort(int *arr, int left, int right);
int partition(int *arr, int left, int right);
void swap(int *a, int *b);
void print_array(int *arr, int size);
double get_time();
int main() {
int *arr = (int *)malloc(ARRAY_SIZE * sizeof(int));
for (int i = 0; i < ARRAY_SIZE; i++) {
arr[i] = rand() % 1000000;
}
double start_time = get_time();
thread_pool_t pool;
init_thread_pool(&pool, THREAD_NUM);
quicksort_args_t args = {
.arr = arr,
.left = 0,
.right = ARRAY_SIZE - 1
};
submit_task(&pool, &args);
join_thread_pool(&pool);
double end_time = get_time();
printf("Sorted array:\n");
print_array(arr, ARRAY_SIZE);
printf("Time elapsed: %f seconds\n", end_time - start_time);
free(arr);
return 0;
}
void init_thread_pool(thread_pool_t *pool, int worker_num) {
pool->worker_num = worker_num;
pool->workers = (worker_t *)malloc(worker_num * sizeof(worker_t));
pool->task_count = 0;
pool->task_index = 0;
pool->done = 0;
pthread_mutex_init(&pool->mutex, NULL);
pthread_cond_init(&pool->cond, NULL);
for (int i = 0; i < worker_num; i++) {
pool->workers[i].args = NULL;
pthread_create(&pool->workers[i].thread, NULL, worker, pool);
}
}
void submit_task(thread_pool_t *pool, quicksort_args_t *args) {
pthread_mutex_lock(&pool->mutex);
pool->task_count++;
pool->workers[pool->task_index].args = args;
pool->task_index = (pool->task_index + 1) % pool->worker_num;
pthread_cond_signal(&pool->cond);
pthread_mutex_unlock(&pool->mutex);
}
void join_thread_pool(thread_pool_t *pool) {
pthread_mutex_lock(&pool->mutex);
while (pool->done == 0) {
pthread_cond_wait(&pool->cond, &pool->mutex);
}
pthread_mutex_unlock(&pool->mutex);
}
void *worker(void *args) {
thread_pool_t *pool = (thread_pool_t *)args;
while (1) {
pthread_mutex_lock(&pool->mutex);
while (pool->task_count == 0 && pool->done == 0) {
pthread_cond_wait(&pool->cond, &pool->mutex);
}
if (pool->done == 1) {
pthread_mutex_unlock(&pool->mutex);
break;
}
quicksort_args_t *task_args = pool->workers[pool->task_index].args;
pool->task_index = (pool->task_index + 1) % pool->worker_num;
pool->task_count--;
pthread_mutex_unlock(&pool->mutex);
quicksort(task_args->arr, task_args->left, task_args->right);
free(task_args);
if (pool->task_count == 0) {
pthread_mutex_lock(&pool->mutex);
if (pool->task_count == 0) {
pool->done = 1;
pthread_cond_signal(&pool->cond);
}
pthread_mutex_unlock(&pool->mutex);
}
}
return NULL;
}
void quicksort(int *arr, int left, int right) {
if (left >= right) {
return;
}
int pivot = partition(arr, left, right);
quicksort(arr, left, pivot - 1);
quicksort(arr, pivot + 1, right);
}
int partition(int *arr, int left, int right) {
int pivot = arr[right];
int i = left - 1;
for (int j = left; j < right; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[right]);
return i + 1;
}
void swap(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
void print_array(int *arr, int size) {
for (int i = 0; i < size; i++) {
printf("%d ", arr[i]);
}
printf("\n");
}
double get_time() {
struct timeval tv;
gettimeofday(&tv, NULL);
return (double)tv.tv_sec + (double)tv.tv_usec / 1000000.0;
}
```
首先在`init_thread_pool`函数中初始化线程池,创建`worker_num`个线程,并将其保存在`pool->workers`数组中。每个线程将执行`worker`函数。
在`submit_task`函数中,我们将任务加入到线程池中。我们使用一个循环数组来记录每个线程的任务,每次调用`submit_task`时,我们将任务保存在下一个线程的任务数组中。`task_count`变量记录了任务数目,`task_index`变量记录了下一个将要保存任务的线程的索引。
在`join_thread_pool`函数中,我们等待所有线程完成任务。`done`变量表示线程池的所有任务是否已经完成。
`worker`函数是每个线程执行的函数。它使用`pthread_cond_wait`函数等待任务,直到线程池中有任务可以执行。然后它获取下一个任务,执行任务,并释放任务的内存。当线程池中没有任务时,它会等待一个信号,直到有新的任务进入线程池或者线程池的所有任务都已经完成。
`quicksort`和`partition`函数是快速排序的实现。`swap`函数用于交换数组中的两个元素。
`print_array`函数用于打印数组。
`get_time`函数用于获取当前时间,用于计算排序的时间。
我们可以在`main`函数中调用`init_thread_pool`函数初始化线程池,创建一个包含`ARRAY_SIZE`个元素的随机整数数组,并将其传递给`quicksort`函数。然后我们调用`submit_task`函数将任务提交到线程池中,并调用`join_thread_pool`函数等待线程池中的所有任务完成。最后我们打印排序后的数组,并计算排序所花费的时间。
阅读全文
相关推荐



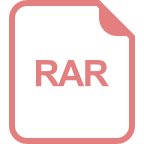











