有一个全局数组int arr[]={1,2,3,4,5,6,7,8,9,10},现需要使用两个线程按顺序交替将数组内容打印出来(每个线程打印一次,直到全部打印完)用c语言写
时间: 2024-10-18 17:10:07 浏览: 12
在C语言中,为了实现这个功能,我们可以使用互斥锁(mutex)和条件变量(condition variable)来同步两个线程。这里是一个简单的示例,假设我们有`pthread.h`库来进行线程操作:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#define ARRAY_SIZE 10
int arr[ARRAY_SIZE] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
pthread_mutex_t mutex; // 互斥锁
pthread_cond_t condition; // 条件变量
void *thread_func(void *arg) {
int index = (int)arg;
while (1) {
pthread_mutex_lock(&mutex); // 获取锁
if (index >= ARRAY_SIZE || index % 2 == 0) { // 线程交替
printf("Thread %d: Printing array element %d\n", index, arr[index]);
index++;
pthread_cond_signal(&condition); // 如果轮到下一个,唤醒另一个线程
} else {
pthread_cond_wait(&condition, &mutex); // 等待条件满足
}
pthread_mutex_unlock(&mutex); // 释放锁
}
}
int main() {
pthread_t thread1, thread2;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&condition, NULL);
// 创建并启动两个线程
pthread_create(&thread1, NULL, thread_func, (void*)0);
pthread_create(&thread2, NULL, thread_func, (void*)(int)1);
// 等待两个线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
// 销毁互斥锁和条件变量
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&condition);
return 0;
}
阅读全文
相关推荐
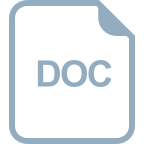
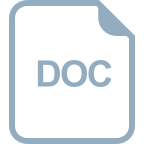
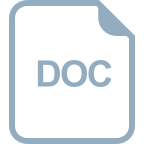















