用c++对一个4位的整数进行加密。加密规则如下:每位数字都加上5 ,然后用和除以10的余数代替该数字,再将第一位和第四位交换,第二位和第三位交换。要求从键盘输入四位数,系统自动给出加密后的数。测试数据至少包含:四位整数,三位整数,五位整数
时间: 2024-11-24 22:40:35 浏览: 12
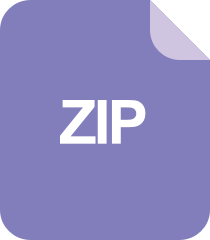
hexl:英特尔同态加密加速库可加速同态加密中使用的模块化算术运算
在C++中,你可以通过以下步骤实现这个简单的4位整数加密过程:
```cpp
#include <iostream>
using namespace std;
int encrypt(int num) {
// 将数字每位加5并取余10
int encrypted[4];
for (int i = 0; i < 4; ++i) {
encrypted[i] = (num % 10 + 5) % 10;
num /= 10;
}
// 交换位置
swap(encrypted[0], encrypted[3]);
swap(encrypted[1], encrypted[2]);
// 拼接成新的4位数
int result = encrypted[0] * 1000 + encrypted[1] * 100 + encrypted[2] * 10 + encrypted[3];
return result;
}
int main() {
// 读取用户输入的四位数
int input;
cout << "请输入一个4位整数:";
cin >> input;
// 加密
int encrypted_num = encrypt(input);
// 输出结果
cout << "加密后的数值是:" << encrypted_num << endl;
// 测试其他情况,如三位数、五位数
int test_cases[] = {987, 12345, 67890};
for (int case_num : test_cases) {
if (case_num >= 1000 && case_num <= 9999) {
cout << "对于测试案例:" << case_num << ", 加密结果是:" << encrypt(case_num) << endl;
} else {
cout << "测试案例:" << case_num << " 无效,因为它不是4位数" << endl;
}
}
return 0;
}
```
这段代码首先定义了一个`encrypt`函数,用于执行加密操作。在`main`函数中,我们获取用户输入的4位数,并调用加密函数。接着,我们提供了一些测试案例,包括3位数和5位数,检查是否满足题目要求。
阅读全文
相关推荐
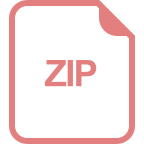
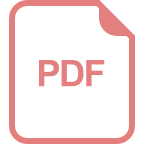








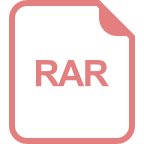
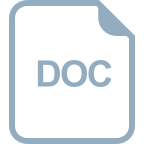
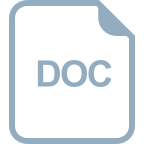
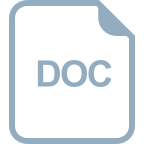
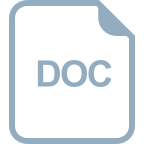
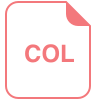

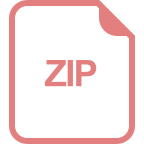