java推箱子的游戏代码
时间: 2024-03-24 11:35:12 浏览: 32
推箱子游戏是一种经典的益智游戏,玩家需要将箱子推到指定位置才能过关。下面是一个简单的Java推箱子游戏的代码示例:
```java
import java.util.Scanner;
public class PushBoxGame {
private static final int WALL = 1;
private static final int BOX = 2;
private static final int TARGET = 3;
private static final int PLAYER = 4;
private static final int EMPTY = 0;
private static final int ROWS = 5;
private static final int COLS = 5;
private static int[][] map = {
{1, 1, 1, 1, 1},
{1, 4, 0, 2, 1},
{1, 0, 0, 3, 1},
{1, 1, 1, 1, 1},
{1, 1, 1, 1, 1}
};
private static int playerRow = 1;
private static int playerCol = 1;
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
while (true) {
printMap();
System.out.println("请输入移动方向(w上,s下,a左,d右):");
String direction = scanner.nextLine();
movePlayer(direction);
if (isWin()) {
System.out.println("恭喜你,游戏胜利!");
break;
}
}
}
private static void printMap() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
switch (map[i][j]) {
case WALL:
System.out.print("# ");
break;
case BOX:
System.out.print("$ ");
break;
case TARGET:
System.out.print("@ ");
break;
case PLAYER:
System.out.print("* ");
break;
case EMPTY:
System.out.print(" ");
break;
}
}
System.out.println();
}
}
private static void movePlayer(String direction) {
int newRow = playerRow;
int newCol = playerCol;
switch (direction) {
case "w":
newRow--;
break;
case "s":
newRow++;
break;
case "a":
newCol--;
break;
case "d":
newCol++;
break;
}
if (map[newRow][newCol] == EMPTY) {
map[playerRow][playerCol] = EMPTY;
map[newRow][newCol] = PLAYER;
playerRow = newRow;
playerCol = newCol;
} else if (map[newRow][newCol] == BOX) {
int nextBoxRow = newRow + (newRow - playerRow);
int nextBoxCol = newCol + (newCol - playerCol);
if (map[nextBoxRow][nextBoxCol] == EMPTY) {
map[playerRow][playerCol] = EMPTY;
map[newRow][newCol] = PLAYER;
map[nextBoxRow][nextBoxCol] = BOX;
playerRow = newRow;
playerCol = newCol; }
}
}
private static boolean isWin() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (map[i][j] == TARGET) {
if (map[i][j] != BOX) {
return false;
}
}
}
}
return true;
}
}
```
这段代码实现了一个简单的5x5的推箱子游戏。玩家通过输入w、s、a、d来控制人物的移动方向,将箱子推到目标位置即可胜利。游戏地图使用二维数组表示,其中1表示墙壁,2表示箱子,3表示目标位置,4表示玩家,0表示空地。
相关推荐












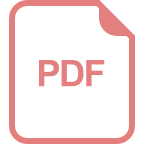