java动态规划解决8皇后问题
时间: 2024-05-07 17:14:12 浏览: 96
根据引用中的描述,八皇后问题可以使用回溯法来解决。当然,也可以使用动态规划。以下是Java动态规划解决八皇后问题的代码示例[^1]:
```java
public class EightQueenDP {
private static final int CHESSBOARD_SIZE = 8;
public static void main(String[] args) {
int[][] result = eightQueen();
printChessboard(result);
}
private static int[][] eightQueen() {
int[][] chessboard = new int[CHESSBOARD_SIZE][CHESSBOARD_SIZE];
int[] queenPos = new int[CHESSBOARD_SIZE];
for (int i = 0; i < CHESSBOARD_SIZE; i++) {
Arrays.fill(chessboard[i], -1);
}
for (int i = 0; i < CHESSBOARD_SIZE; i++) {
int max = -1;
int row = -1;
for (int j = 0; j < CHESSBOARD_SIZE; j++) {
if (queenPos[j] == 0) {
int count = calculateAttackCount(chessboard, i, j);
if (count > max) {
max = count;
row = j;
}
}
}
chessboard[i][row] = 1;
queenPos[row] = 1;
}
return chessboard;
}
private static int calculateAttackCount(int[][] chessboard, int row, int col) {
int count = 0;
for (int i = row - 1; i >= 0; i--) {
if (chessboard[i][col] == 1) {
count++;
}
if (col - row + i >= 0 && chessboard[i][col - row + i] == 1) {
count++;
}
if (col + row - i < CHESSBOARD_SIZE && chessboard[i][col + row - i] == 1) {
count++;
}
}
return count;
}
private static void printChessboard(int[][] chessboard) {
for (int i = 0; i < CHESSBOARD_SIZE; i++) {
for (int j = 0; j < CHESSBOARD_SIZE; j++) {
if (chessboard[i][j] == 1) {
System.out.print("Q ");
} else {
System.out.print("* ");
}
}
System.out.println();
}
}
}
```
这个方法使用了一个二维数组来表示棋盘,1表示该位置有皇后,-1表示该位置没有皇后。使用一维数组queenPos来表示每列是否已经放置了皇后。在每一行中,先计算每个空位置的攻击数,然后选择攻击数最大的位置来放置皇后,直到所有皇后都被放置。
阅读全文
相关推荐

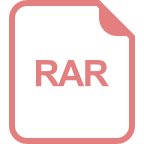











