用STM32F103写一个计算器
时间: 2023-11-17 08:07:11 浏览: 132
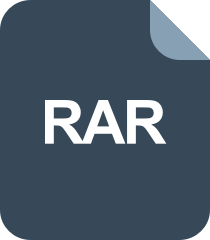
STM32做计算器
写一个计算器需要涉及到很多方面,包括硬件和软件。在STM32F103上实现一个计算器需要以下步骤:
1. 硬件设计:需要设计一个电路板,连接按键、显示屏和STM32F103等器件。按键用来输入数字和运算符,显示屏用来显示计算结果。
2. 软件开发:需要编写嵌入式软件,实现计算器的各个功能。主要包括按键扫描、数字和运算符的输入、运算符优先级处理、计算结果的显示等。
下面是一个简单的计算器程序示例。该程序支持加减乘除四则运算,可以通过按键输入数字和运算符,支持连续计算。
```c
#include "stm32f10x.h"
#include "stm32f10x_gpio.h"
#include "stm32f10x_rcc.h"
#include "stm32f10x_usart.h"
#include "stdio.h"
#define KEY0 GPIO_Pin_0
#define KEY1 GPIO_Pin_1
#define KEY2 GPIO_Pin_2
#define KEY3 GPIO_Pin_3
#define KEY_PORT GPIOA
void GPIO_Configuration(void);
void USART_Configuration(void);
void USART_SendString(char* str);
void delay_us(u32 nus);
void delay_ms(u16 nms);
void Key_Init(void);
int main(void)
{
u8 key = 0;
u8 num1 = 0, num2 = 0, op = 0;
int result = 0;
char buffer[16];
GPIO_Configuration();
USART_Configuration();
Key_Init();
while (1)
{
if (GPIO_ReadInputDataBit(KEY_PORT, KEY0) == 0)
{
key = 1;
USART_SendString("1");
delay_ms(50);
}
if (GPIO_ReadInputDataBit(KEY_PORT, KEY1) == 0)
{
key = 2;
USART_SendString("2");
delay_ms(50);
}
if (GPIO_ReadInputDataBit(KEY_PORT, KEY2) == 0)
{
key = 3;
USART_SendString("3");
delay_ms(50);
}
if (GPIO_ReadInputDataBit(KEY_PORT, KEY3) == 0)
{
key = 4;
USART_SendString("4");
delay_ms(50);
}
if (key >= 1 && key <= 9)
{
if (op == 0)
{
num1 = num1 * 10 + key;
}
else
{
num2 = num2 * 10 + key;
}
}
else if (key == 10)
{
op = 1;
}
else if (key == 11)
{
op = 2;
}
else if (key == 12)
{
op = 3;
}
else if (key == 13)
{
op = 4;
}
else if (key == 14)
{
switch (op)
{
case 1:
result = num1 + num2;
break;
case 2:
result = num1 - num2;
break;
case 3:
result = num1 * num2;
break;
case 4:
if (num2 == 0)
{
USART_SendString("Error");
}
else
{
result = num1 / num2;
}
break;
default:
break;
}
sprintf(buffer, "=%d", result);
USART_SendString(buffer);
num1 = result;
num2 = 0;
op = 0;
}
key = 0;
}
}
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void USART_Configuration(void)
{
USART_InitTypeDef USART_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
USART_Cmd(USART1, ENABLE);
}
void USART_SendString(char* str)
{
while (*str != '\0')
{
USART_SendData(USART1, (uint16_t) *str++);
while (USART_GetFlagStatus(USART1, USART_FLAG_TXE) == RESET);
}
}
void delay_us(u32 nus)
{
u32 i;
for (i = 0; i < nus; i++);
}
void delay_ms(u16 nms)
{
u16 i, j;
for (i = 0; i < nms; i++)
{
for (j = 0; j < 1141; j++);
}
}
void Key_Init(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = KEY0 | KEY1 | KEY2 | KEY3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(KEY_PORT, &GPIO_InitStructure);
}
```
该程序实现了一个简单的四则运算计算器,通过串口输出运算结果。需要注意的是,该程序只是一个示例,还有很多需要完善和优化的地方。
阅读全文
相关推荐



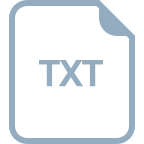
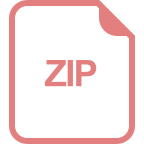




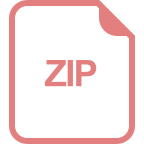
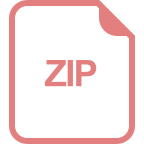
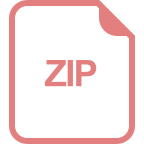
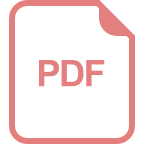
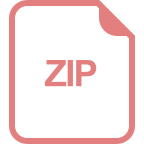
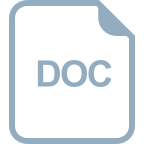