c# MemoryStream 导致内存溢出
时间: 2023-10-28 18:34:55 浏览: 470
C# 的 MemoryStream 类是一个非常方便的类,用于在内存中创建和操作流。但是,如果不小心使用它,可能会导致内存溢出。
常见的内存溢出情况是在写入大量数据时,内存使用量增加,直到达到计算机内存的极限。这种情况下,程序可能会崩溃或变得非常缓慢。
为了避免这种情况,可以考虑以下几点:
1. 使用指定大小的缓冲区。在实例化 MemoryStream 对象时,可以指定一个缓冲区大小,以便限制内存使用量。
2. 不要在内存不足的情况下继续写入数据。可以使用 CanWrite 属性检查是否可以继续写入数据。
3. 在使用完 MemoryStream 后,一定要调用 Dispose() 方法,以释放占用的内存资源。
4. 考虑使用其他类型的流,如 FileStream,以便将数据写入磁盘上的文件,而不是在内存中。
总之,使用 MemoryStream 时要小心,确保正确使用和释放内存资源,以避免内存溢出。
相关问题
c# 图片压缩内存溢出
图片压缩时可能会出现内存溢出的问题,特别是当处理大型图片时。为了避免这个问题,可以尝试使用以下方法:
1. 逐行读取:不要一次性读取整张图片,而是按行读取,然后逐行进行处理。这样可以降低内存占用。
2. 使用流:可以使用 MemoryStream 来读取和写入图片数据,这样可以避免频繁的文件读写操作。
3. 调整压缩质量:压缩质量越高,生成的图片文件越大,占用的内存也越多。因此,可以适当调整压缩质量,来减小内存占用。
4. 使用 using 语句:在使用完 Bitmap、Graphics 和 Image 对象后,应该及时释放它们所占用的资源。可以使用 using 语句来自动释放资源,以避免内存泄漏。
下面是一个示例代码,演示如何使用流来进行图片压缩:
```csharp
public static void CompressImage(string sourcePath, string destinationPath, int maxWidth, int maxHeight, int quality)
{
using (var sourceStream = new FileStream(sourcePath, FileMode.Open, FileAccess.Read))
{
using (var sourceImage = Image.FromStream(sourceStream))
{
int sourceWidth = sourceImage.Width;
int sourceHeight = sourceImage.Height;
// 计算压缩比例
double ratio = Math.Min((double)maxWidth / sourceWidth, (double)maxHeight / sourceHeight);
int newWidth = (int)(sourceWidth * ratio);
int newHeight = (int)(sourceHeight * ratio);
// 创建新图片
using (var bitmap = new Bitmap(newWidth, newHeight))
{
using (var graphics = Graphics.FromImage(bitmap))
{
// 设置画布的质量
graphics.InterpolationMode = System.Drawing.Drawing2D.InterpolationMode.HighQualityBicubic;
graphics.SmoothingMode = System.Drawing.Drawing2D.SmoothingMode.HighQuality;
graphics.PixelOffsetMode = System.Drawing.Drawing2D.PixelOffsetMode.HighQuality;
graphics.CompositingQuality = System.Drawing.Drawing2D.CompositingQuality.HighQuality;
// 绘制新图片
graphics.DrawImage(sourceImage, new Rectangle(0, 0, newWidth, newHeight));
}
// 保存新图片
using (var destinationStream = new FileStream(destinationPath, FileMode.Create))
{
var encoderParams = new EncoderParameters(1);
encoderParams.Param[0] = new EncoderParameter(Encoder.Quality, quality);
var jpegEncoder = ImageCodecInfo.GetImageEncoders().FirstOrDefault(x => x.FormatID == ImageFormat.Jpeg.Guid);
bitmap.Save(destinationStream, jpegEncoder, encoderParams);
}
}
}
}
}
```
在这个方法中,我们使用 FileStream 来读取源图片,使用 MemoryStream 来写入新图片,使用 using 语句来自动释放资源。同时,我们还可以通过调整 quality 参数来控制压缩质量。
c# 从本地读取一张大图,报警内存溢出
在C#中,直接通过`Bitmap`或`Image`类的构造函数读取大尺寸图片可能会导致内存溢出,因为这些类会在内存中完全加载整个图像。特别是如果你尝试一次性将大图片读入到PictureBox控件中,如果图片太大,系统无法一次性容纳其所有内容,就会报内存溢出错误。
为了避免这种情况,你可以采用以下策略:
1. **逐块加载**:
使用`Bitmap`的`LockBits`方法,它可以让你以“锁定”(lock)的方式访问位图的数据,只加载你需要的部分。每次只读取并释放一小部分,直到全部加载完毕。
```csharp
using (var img = new Bitmap(filePath))
{
int bytesPerPixel = img.PixelFormat == PixelFormat.Format32bppArgb ? 4 : 2; // 根据图像格式计算每像素字节数
int imageWidth = img.Width;
int heightInBlocks = Math.Min(height / blockSize, maxBlocks); // 分割高度为大小为blockSize的块
for (int y = 0; y < heightInBlocks; y++)
{
Rectangle rect = new Rectangle(0, y * blockSize, imageWidth, blockSize);
using (var data = img.LockBits(rect, ImageLockMode.ReadOnly, img.PixelFormat))
{
byte* ptr = (byte*)data.Scan0;
// 在这里处理和显示每个块的像素数据...
}
}
}
```
2. **流式处理**:
可以使用`Image.FromStream`或`MemoryStream`配合`StreamReader`,以小块的形式从文件流中读取图像,减少内存消耗。
```csharp
using (FileStream stream = File.OpenRead(filePath))
using (MemoryStream ms = new MemoryStream())
{
byte[] buffer = new byte[BufferSize];
int bytesRead;
while ((bytesRead = stream.Read(buffer, 0, BufferSize)) > 0)
{
ms.Write(buffer, 0, bytesRead);
// 读取到的这部分数据可以直接用于创建新的Bitmap实例或其他操作
}
Image image = Image.FromStream(ms);
}
```
以上方法能有效地防止内存溢出,不过也取决于你的应用对实时显示的需求程度。
阅读全文
相关推荐

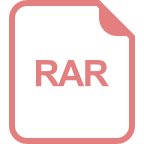













