vmware虚拟机root用户
时间: 2023-10-12 17:21:22 浏览: 35
好的,这是一个题目和代码相关的问题,可以直接回答。
以下是实现创建链表、打印链表、交换元素、展示链表、销毁链表的代码:
```
#include <stdio.h>
#include <stdlib.h>
typedef struct Node{
int data;
struct Node *next;
}Node;
// 创建链表
Node* createList(int* L, int n){
Node *head, *tail, *newNode;
head = NULL;
for(int i=0; i<n; i++){
newNode = (Node*)malloc(sizeof(Node));
newNode->data = L[i];
newNode->next = NULL;
if(head == NULL){
head = newNode;
tail = newNode;
}else{
tail->next = newNode;
tail = newNode;
}
}
return head;
}
// 打印链表
void printList(Node* head){
while(head != NULL){
printf("%d ", head->data);
head = head->next;
}
printf("\n");
}
// 交换链表中的两个元素
void swap(Node* p1, Node* p2){
int temp = p1->data;
p1->data = p2->data;
p2->data = temp;
}
// 展示链表,并交换以第一个元素为分界线的元素
void displayList(Node* head){
int base = head->data;
Node *p1 = head, *p2 = head;
while(p2 != NULL){
if(p2->data < base){
swap(p1, p2);
p1 = p1->next;
}
p2 = p2->next;
}
printList(head);
}
// 销毁链表
void destroyList(Node* head){
Node *p = head, *q;
while(p != NULL){
q = p->next;
free(p);
p = q;
}
}
int main(){
int L[] = {5, 2, 7, 8, 1, 0, 3, 6, 9, 4};
int n = sizeof(L) / sizeof(int);
Node* head = createList(L, n);
printf("原始链表:\n");
printList(head);
printf("交换元素后的链表:\n");
displayList(head);
printf("销毁链表...\n");
destroyList(head);
return 0;
}
```
希望我的回答能解决你的问题。
相关推荐
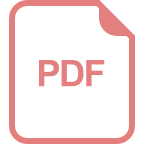
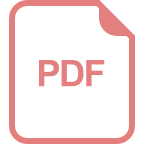
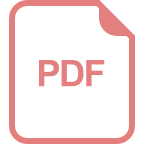














