如何用Python表示求和和连乘
时间: 2023-09-20 21:05:54 浏览: 74
### 回答1:
使用Python可以通过内置函数 `sum()` 表示求和操作,例如:
```python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print(total) # 输出结果为 15
```
其中,`sum()` 函数的参数为一个可迭代对象,例如列表、元组、集合等。
而连乘可以通过内置函数 `reduce()` 表示,需要导入 `functools` 模块才能使用,例如:
```python
from functools import reduce
numbers = [1, 2, 3, 4, 5]
product = reduce(lambda x, y: x * y, numbers)
print(product) # 输出结果为 120
```
其中,`reduce()` 函数的第一个参数为一个函数,该函数接受两个参数并返回一个值,表示对可迭代对象进行归约操作。在本例中,使用匿名函数 `lambda` 实现了两数相乘的操作。`reduce()` 函数的第二个参数为可迭代对象,表示需要进行归约操作的数列。
### 回答2:
在Python中,可以使用循环结构来表示求和和连乘。
求和可以通过使用for循环结合累加器来实现。首先,我们需要定义一个变量来存储累加的结果,通常称为累加器变量。然后,我们可以使用for循环来遍历需要求和的数列或范围,并将每个元素依次累加到累加器变量中。最后,输出累加器变量的值,即为求和的结果。例如,我们可以使用以下代码表示求和:
```python
# 定义一个包含需要求和的数列
numbers = [1, 2, 3, 4, 5]
# 定义累加器变量
sum_result = 0
# 使用for循环累加求和
for num in numbers:
sum_result += num
# 输出求和结果
print("求和结果:", sum_result)
```
连乘可以通过类似的方式使用for循环来实现。首先,我们需要定义一个变量来存储累乘的结果,通常称为累乘器变量。然后,我们可以使用for循环来遍历需要连乘的数列或范围,并将每个元素依次累乘到累乘器变量中。最后,输出累乘器变量的值,即为连乘的结果。例如,我们可以使用以下代码表示连乘:
```python
# 定义一个包含需要连乘的数列
numbers = [1, 2, 3, 4, 5]
# 定义累乘器变量
product_result = 1
# 使用for循环累乘连乘
for num in numbers:
product_result *= num
# 输出连乘结果
print("连乘结果:", product_result)
```
通过这样的方式,我们可以使用Python来表示求和和连乘,并得到相应的结果。
### 回答3:
要用Python表示求和,可以使用for循环或者使用内置函数sum()。下面是使用for循环的示例代码:
```python
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
print("求和结果为:", total)
```
另外,使用sum()函数也可以实现:
```python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
print("求和结果为:", total)
```
要用Python表示连乘,可以使用for循环或者使用math库中的函数。下面是使用for循环的示例代码:
```python
numbers = [1, 2, 3, 4, 5]
total = 1
for num in numbers:
total *= num
print("连乘结果为:", total)
```
另外,也可以使用math库中的函数来实现:
```python
import math
numbers = [1, 2, 3, 4, 5]
total = math.prod(numbers)
print("连乘结果为:", total)
```
以上是使用Python来表示求和和连乘的方法。无论是使用for循环还是使用内置函数,都可以很方便地计算出结果。
相关推荐
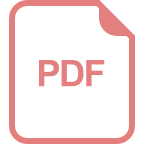
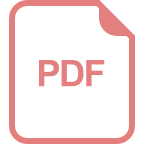
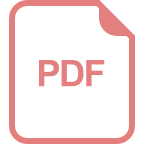
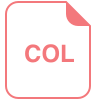













