编写Java应用程序,创建一个可序列化类,将该类的对象写入到文件中。用对象输入流读取并显示对象信息。
时间: 2024-03-19 11:44:30 浏览: 56
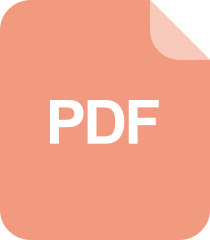
Java将对象保存到文件中/从文件中读取对象的方法
好的,我可以帮你解答这个问题。以下是一个示例程序,它演示了如何创建一个可序列化类,将该类的对象写入到文件中,然后使用对象输入流读取并显示对象信息。你可以参考这个程序来编写自己的Java应用程序。
```java
import java.io.*;
public class SerializableDemo {
public static void main(String[] args) {
// 创建一个可序列化的对象
Person person = new Person("Tom", 18);
// 将对象写入到文件中
try {
FileOutputStream fos = new FileOutputStream("person.ser");
ObjectOutputStream oos = new ObjectOutputStream(fos);
oos.writeObject(person);
oos.close();
fos.close();
System.out.println("Serialized data is saved in person.ser");
} catch (IOException e) {
e.printStackTrace();
}
// 使用对象输入流读取并显示对象信息
try {
FileInputStream fis = new FileInputStream("person.ser");
ObjectInputStream ois = new ObjectInputStream(fis);
Person p = (Person) ois.readObject();
ois.close();
fis.close();
System.out.println("Deserialized data: ");
System.out.println("Name: " + p.getName());
System.out.println("Age: " + p.getAge());
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
}
// 可序列化的类
class Person implements Serializable {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
}
```
在这个程序中,我们定义了一个可序列化的类 `Person`,它有两个属性:`name` 和 `age`。我们创建了一个 `Person` 对象,并将它写入到文件 `person.ser` 中。然后,我们使用对象输入流读取 `person.ser` 文件,并显示 `Person` 对象的信息。
注意,为了能够序列化和反序列化一个对象,该对象所属的类必须实现 `Serializable` 接口。在上面的程序中,我们让 `Person` 类实现了 `Serializable` 接口,这样就可以将 `Person` 对象序列化和反序列化了。
希望这个示例程序能够对你有所帮助!
阅读全文
相关推荐
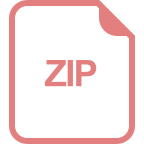
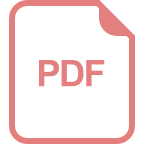





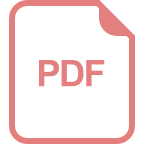
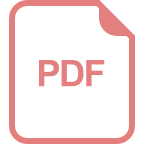
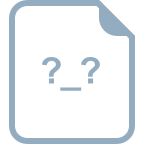
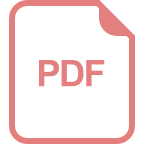
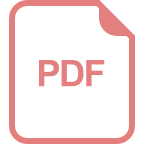
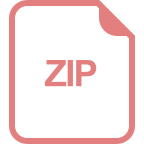
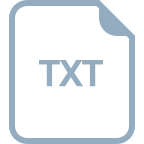
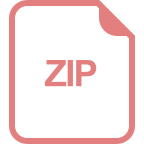