变步长梯形方法、变步Simpson方法和Romberg方法求积分的matlab代码
时间: 2024-12-15 22:29:29 浏览: 12
变步长梯形法、变步Simpson方法和Romberg积分方法都是数值积分技术,用于近似函数的定积分。在MATLAB中,你可以通过内建函数`quadl`和一些自定义步骤来实现这些方法。下面是一些基本的示例:
1. **变步长梯形法 (Trapezoidal Rule with Variable Steps)**
```matlab
function integral = trapezoidal(f, a, b, n)
h = (b - a) / n;
sum_area = f(a) + f(b);
for i = 1:(n-1)
x = a + (i + 0.5) * h;
sum_area += f(x);
end
integral = sum_area * h; % 经过修改后的面积总和作为近似值
end
% 使用示例
f = @(x) x^2; % 定义你要积分的函数
integral_approx = trapezoidal(f, 0, 1, 10); % 求解区间[0,1]的积分,用10等分
```
2. **变步Simpson's Rule (Simpson's Rule with Variable Steps)**
```matlab
function integral = simpson(f, a, b, n)
h = (b - a) / n;
if mod(n, 2) == 1 % 如果步数奇数,需要添加中间点
c = a + h / 2;
sum_area = f(a) + 4*f(c) + f(b);
else
sum_area = 2 * (f(a) + f(b)) + 4 * sum(f(a+h*i) for i=1:n/2); % 偶数步数
end
integral = (h / 3) * sum_area; % Simpson公式计算积分
end
integral_approx_simpson = simpson(f, 0, 1, 8); % 使用8等分
```
3. **Romberg Integration (Romberg Method)**
```matlab
function integral = romberg(f, a, b, tol)
R = zeros(0, 2); % 初始化Romberg矩阵
R(1, :) = [1; (b-a)/2];
for n = 2:log2(1e5) % 高精度迭代,直到达到误差容忍度
Tn = zeros(n, 2);
for k = 1:(2^n-1)
Tn(k+1, :) = [Tn(k, :); (f(a+k*(b-a)/(2^n)) + f(a+(k+1)*(b-a)/(2^n))) / 2];
end
R(:, n+1) = R(:, n) + ((2^(n+1)-1)/(2*n+1)) * (Tn(:, 2:end) - Tn(:, 1:end-1));
if norm(R(:, n+1) - R(:, n), inf) < tol
break;
end
end
integral = R(end, 2);
end
integral_romberg = romberg(@(x) x.^2, 0, 1, 1e-6); % 用1e-6作为误差容忍度
```
阅读全文
相关推荐
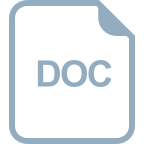
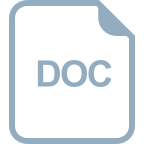
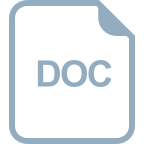


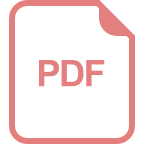
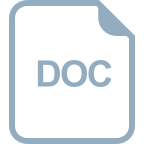
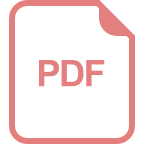
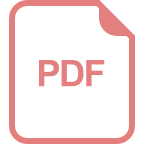
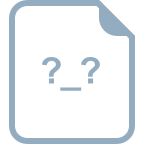
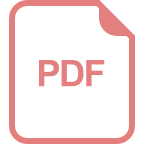
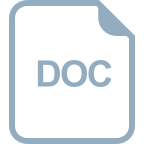
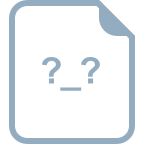
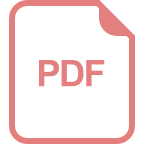
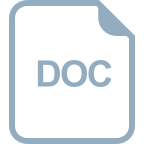
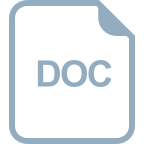
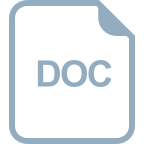
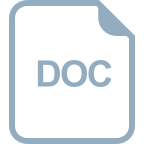
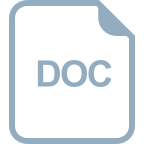