用变步长梯形方法、变步Simpson方法和Romberg方法计算积分的matlab代码
时间: 2024-12-15 16:22:05 浏览: 36
在MATLAB中,你可以使用`integral`函数结合循环结构来实现变步长梯形法、变步长Simpson法和Romberg积分法。下面是一些基本示例:
1. 变步长梯形法(Trapzoidal Rule):
```matlab
function trapezoidal(f, a, b, n)
h = (b - a) / n;
trapz = (f(a) + f(b)) * h / 2; % 梯形面积公式
for i = 1:n-1
xi = a + i * h;
trapz += f(xi);
end
disp(['Trapezoidal approximation: ', num2str(trapz)]);
end
```
呼叫方式: `trapezoidal(@(x)x.^2, 0, 1, 10);`
2. 变步长辛普森法则(Simpson's Rule):
```matlab
function simpson(f, a, b, n)
h = (b - a) / n;
if mod(n, 2) == 0
warning('Odd number of intervals required for Simpson''s rule.');
end
simpson_sum = f(a) + f(b);
for i = 1:2:(n-1)
xi = a + i * h;
simpson_sum += 4 * f(xi);
end
for i = 2:2:(n-2)
xi = a + i * h;
simpson_sum += 2 * f(xi);
end
disp(['Simpson''s approximation: ', num2str(simpson_sum * h / 3)]);
end
```
呼叫方式: `simpson(@(x)x.^3, 0, 1, 10);`
3. Romberg积分法(Romberg Integration):
```matlab
function romberg(f, a, b, tol)
R = zeros(2, 1);
R(1, 1) = (b - a) * (f(a) + f(b))/2;
for n = 2:2:100 % 可调整精度等级
m = floor((n - 1) / 2);
for j = m:-1:1
R(n, 1) = R(n, 1) + (R(2*j+1, 1) - R(2*j-1, 1)) / (4^j);
end
error = abs(R(n, 1) - R(n-1, 1));
if error < tol
break;
end
end
disp(['Romberg approximation: ', num2str(R(n, 1))]);
end
```
呼叫方式: `romberg(@(x)x.^4, 0, 1, 1e-6);`
阅读全文
相关推荐
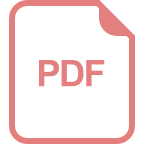
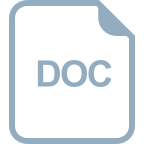
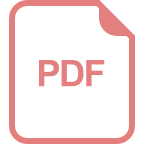















