编写Python代码,分别用复化梯形公式、复化Simpson公式和Romberg公式计算给定函数的数值积分
时间: 2024-05-01 11:21:30 浏览: 101
下面是一个示例代码,其中给定了函数f(x)和积分区间[a,b],可以根据需要修改:
```python
import math
# 定义积分函数
def f(x):
return math.sin(x)
# 定义复化梯形公式
def composite_trapezoidal(f, a, b, n):
h = float(b - a) / n
s = 0.5 * (f(a) + f(b))
for i in range(1, n):
s += f(a + i*h)
return h * s
# 定义复化Simpson公式
def composite_simpson(f, a, b, n):
h = float(b - a) / n
s = f(a) + f(b)
for i in range(1, n):
if i % 2 == 0:
s += 2 * f(a + i*h)
else:
s += 4 * f(a + i*h)
return h * s / 3
# 定义Romberg公式
def romberg(f, a, b, nmax, eps):
R = [[0] * (nmax+1) for _ in range(nmax+1)]
h = b - a
R[0][0] = 0.5 * h * (f(a) + f(b))
for n in range(1, nmax+1):
h *= 0.5
s = 0
for i in range(1, 2**n, 2):
s += f(a + i*h)
R[n][0] = 0.5 * R[n-1][0] + s * h
for m in range(1, n+1):
R[n][m] = (4**m * R[n][m-1] - R[n-1][m-1]) / (4**m - 1)
if abs(R[n][n-1] - R[n][n]) < eps:
break
return R[n][n]
# 测试
a, b = 0, math.pi/2
n = 100
print("复化梯形公式:", composite_trapezoidal(f, a, b, n))
print("复化Simpson公式:", composite_simpson(f, a, b, n))
print("Romberg公式:", romberg(f, a, b, nmax=10, eps=1e-6))
```
其中,`composite_trapezoidal()`、`composite_simpson()`和`romberg()`分别计算复化梯形公式、复化Simpson公式和Romberg公式的数值积分结果。在测试部分,我们给定了函数f(x)=sin(x)和积分区间[0,pi/2],并且设定了n=100,即将积分区间分成100个小区间,然后分别使用三种方法计算积分结果并输出。
阅读全文
相关推荐
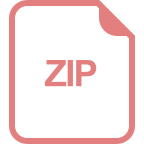
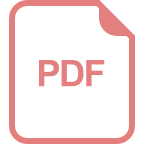
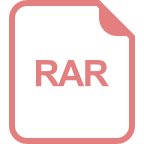















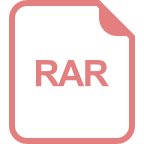