有一链式结构,定义如下 : struct stu{ char name[20]; int no; struct stu *next; }; 创建一个函数create(),实现无头结点的链表,有m个结点,函数的返回值为链表的头指针。 函数接口定义: 在这里描述函数接口。例如: struct stu * create() 在这里解释接口参数。在函数中输入m,及m行数据,完成链表的创建。 裁判测试程序样例: 在这里给出函数被调用进行测试的例子。例如: #include <stdio.h> #include <stdlib.h> struct stu{ char name[20]; int no; struct stu *next;}; struct stu * create(); void show(struct stu *p){ while(p){ printf("%s %d\n",p->name,p->no); p=p->next; } } int main() { struct stu *p=NULL; p=create(); show(p); return 0; } /* 请在这里填写答案 */ 输入样例: 在这里给出一组输入。例如: 2 liming 23 maming 35
时间: 2024-03-17 21:47:15 浏览: 52
以下是实现无头结点的链表,有m个结点的函数create()的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stu{
char name[20];
int no;
struct stu *next;
};
struct stu * create() {
int m;
scanf("%d", &m);
struct stu *head = NULL, *p = NULL;
for (int i = 0; i < m; i++) {
struct stu *node = (struct stu *)malloc(sizeof(struct stu));
scanf("%s %d", node->name, &node->no);
if (head == NULL) {
head = node;
} else {
p->next = node;
}
p = node;
}
if (p != NULL) {
p->next = NULL;
}
return head;
}
```
在主函数中,可以通过调用create()函数来创建链表,并且将返回值赋给指向链表头结点的指针p。然后,可以通过调用show()函数来输出链表中的内容。
完整的程序如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct stu{
char name[20];
int no;
struct stu *next;
};
struct stu * create() {
int m;
scanf("%d", &m);
struct stu *head = NULL, *p = NULL;
for (int i = 0; i < m; i++) {
struct stu *node = (struct stu *)malloc(sizeof(struct stu));
scanf("%s %d", node->name, &node->no);
if (head == NULL) {
head = node;
} else {
p->next = node;
}
p = node;
}
if (p != NULL) {
p->next = NULL;
}
return head;
}
void show(struct stu *p) {
while (p) {
printf("%s %d\n", p->name, p->no);
p = p->next;
}
}
int main() {
struct stu *p = NULL;
p = create();
show(p);
return 0;
}
```
输入样例:
```
2
liming 23
maming 35
```
输出样例:
```
liming 23
maming 35
```
阅读全文
相关推荐
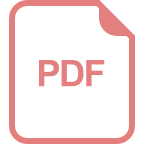
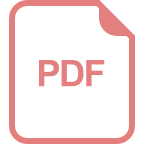
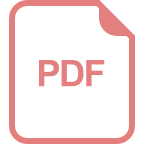







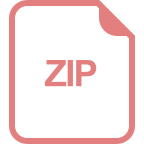
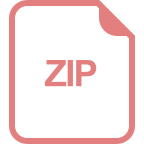
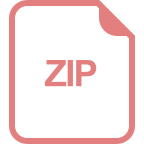
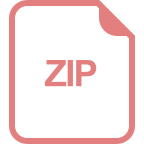
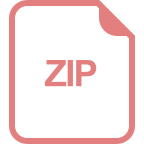
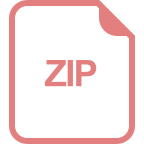