In your own words, explain, using an example, how the this reference works. What is an advantage of using this reference?
时间: 2024-03-14 15:46:54 浏览: 81
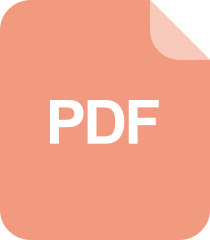
The.MIT.Press.Once.Upon.an.Algorithm.How.Stories.Explain.Computing.0262036630
In object-oriented programming, the `this` reference is used to refer to the current instance of a class. It allows us to access and modify the properties and methods of the current object.
For example, let's say we have a class called `Person` with two properties: `name` and `age`. We can define a method called `introduce` that prints out a message introducing the person with their name and age:
```
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
introduce() {
console.log(`Hi, my name is ${this.name} and I am ${this.age} years old.`);
}
}
let john = new Person("John", 30);
john.introduce(); // Output: Hi, my name is John and I am 30 years old.
```
In this example, `this` refers to the current instance of the `Person` class, which is `john`. By using `this.name` and `this.age`, we can access the properties of the current instance and print them out in the `introduce` method.
An advantage of using the `this` reference is that it allows us to write more flexible and reusable code. If we create multiple instances of the `Person` class with different names and ages, the `introduce` method will automatically adapt and print out the correct information for each instance. Additionally, if we were to rename the `name` or `age` property in the future, we would only need to update it in one place (inside the class), and all methods that use `this.name` or `this.age` would still work correctly.
阅读全文
相关推荐
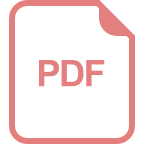
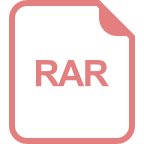

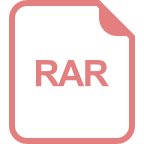
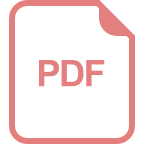
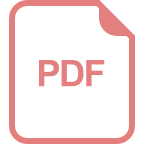
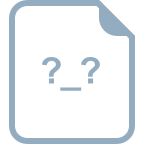
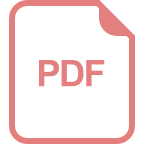
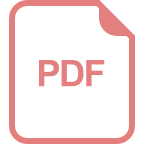
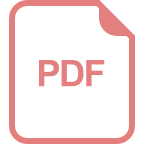
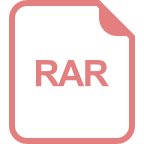
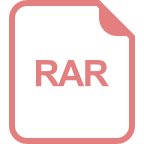
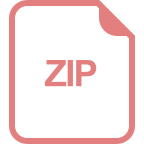