可以跟我说一下在yolov5中融合bifpn的代码改进吗?
时间: 2024-02-09 19:12:31 浏览: 38
BiFPN 是一种用于特征金字塔融合的方法,可以用于目标检测等任务。目前 YOLOv5 的代码中并没有内置的 BiFPN 模块,如果您想在 YOLOv5 中使用 BiFPN,需要对代码进行修改和重新训练。
以下是一个简单的方案来实现 YOLOv5 + BiFPN,仅供参考:
1.准备数据
首先需要准备好用于训练和测试的数据集。
2. 确定网络结构
在 YOLOv5 的 backbone 中添加一些额外的卷积层,以产生不同尺度和分辨率的特征图。具体来说,可以在 CSPDarknet53 的顶部添加几个 Conv 层,并使用这些层的输出作为 BiFPN 模块的输入。在这里,我们添加了 3 个 Conv 层,分别产生 80x80、40x40 和 20x20 的特征图。
```python
import torch.nn as nn
from models.common import Conv
class BiFPN(nn.Module):
def __init__(self, channels):
super(BiFPN, self).__init__()
self.channels = channels
self.conv6_up = Conv(channels, channels, 1, 1)
self.conv5_up = Conv(channels, channels, 1, 1)
self.conv4_up = Conv(channels, channels, 1, 1)
self.conv3_up = Conv(channels, channels, 1, 1)
self.conv4_down = Conv(channels, channels, 1, 1)
self.conv5_down = Conv(channels, channels, 1, 1)
self.conv6_down = Conv(channels, channels, 1, 1)
self.conv7_down = Conv(channels, channels, 1, 1)
```
3. 实现 BiFPN 模块
在这里,我们使用一个由多个 Conv 层和 BN 层组成的 BiFPN 模块,用于将不同尺度和分辨率的特征图融合在一起。具体来说,我们首先使用 Conv 层将特征图的通道数调整为指定的 channels,然后使用 BN 层对特征进行归一化。
```python
def forward(self, inputs):
c3, c4, c5, c6, c7 = inputs
# Top-down pathway
p6 = self.conv6_up(c6)
p5 = self.conv5_up(c5)
p4 = self.conv4_up(c4)
p3 = self.conv3_up(c3)
# Bottom-up pathway
p4_down = self.conv4_down(p4) + nn.functional.interpolate(p5, scale_factor=2, mode='nearest')
p5_down = self.conv5_down(p5) + nn.functional.interpolate(p6, scale_factor=2, mode='nearest')
p6_down = self.conv6_down(p6) + nn.functional.interpolate(c7, scale_factor=2, mode='nearest')
p3_down = self.conv7_down(p3) + nn.functional.interpolate(p4, scale_factor=2, mode='nearest')
# Combine features
p5 = (p5 + p4_down) / 2
p4 = (p4 + p5_down + p3_down) / 3
p6 = (p6 + p5_down + p6_down) / 3
p7 = (p6_down + p3_down) / 2
return [p3, p4, p5, p6, p7]
```
4. 修改 YOLOv5 的代码
在 YOLOv5 的代码中,我们需要将 BiFPN 模块添加到 backbone 中,然后使用其输出来替换原来的特征图。具体来说,我们可以在 model.py 中的 CSPDarknet 类中添加 BiFPN 模块,并在 forward 方法中使用其输出。
```python
class CSPDarknet(nn.Module):
def __init__(self, input_channels=3, depth=1.0, width=1.0):
super().__init__()
channels = int(64 * width)
self.conv1 = Conv(input_channels, channels, kernel_size=3, stride=1)
self.stages = nn.ModuleList([
CSPStage(channels, channels * 2, int(depth * 3), width),
CSPStage(channels * 2, channels * 4, int(depth * 9), width),
CSPStage(channels * 4, channels * 8, int(depth * 9), width),
CSPStage(channels * 8, channels * 16, int(depth * 3), width),
BiFPN(channels * 16), # Add BiFPN module here
])
self.out_channels = [channels * 2, channels * 4, channels * 8, channels * 16, channels * 16]
self.depth = depth
def forward(self, x):
x = self.conv1(x)
x = self.stages[0](x)
x = self.stages[1](x)
x3 = self.stages[2](x)
x4 = self.stages[3](x3)
x5, x6, x7 = self.stages[4]([x3, x4, x5, x6, x7]) # Use BiFPN output here
return [x3, x4, x5, x6, x7]
```
5. 训练模型
对修改后的代码进行训练,可以使用类似 YOLOv5 官方的训练脚本进行训练。在训练过程中,可以使用验证集评估模型的性能。
以上是一个简单的方案来实现 YOLOv5 + BiFPN,具体实现可能会有所不同,需要根据实际情况进行调整。如果您想详细了解如何实现,建议查阅相关论文和代码,以及咨询深度学习领域的专业人士。
相关推荐
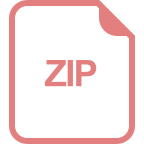
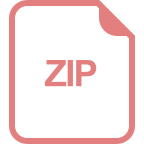
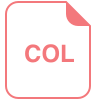
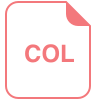
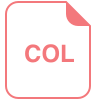
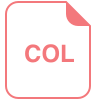
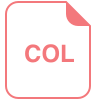









