echarts日历热力图piecewise如何修改最外层边框颜色
时间: 2024-03-11 11:47:40 浏览: 18
要修改echarts日历热力图中piecewise组件最外层边框的颜色,需要在图表的option中对visualMap进行配置。具体操作如下:
1. 找到visualMap配置项,设置piecewise中的borderColor属性。
```javascript
visualMap: {
type: 'piecewise',
min: 0,
max: 100,
left: 'center',
top: 'middle',
show: true,
pieces: [{min: 0, max: 20}, {min: 20, max: 40}, {min: 40, max: 60}, {min: 60, max: 80}, {min: 80, max: 100}],
inRange: {
color: ['#FFFFFF', '#FF4500']
},
borderColor: 'red' // 设置边框线颜色
}
```
2. 重新加载图表。
```javascript
myChart.setOption(option);
```
以上就是修改echarts日历热力图中piecewise组件最外层边框颜色的方法。
相关问题
echarts日历热力图代码
好的,以下是一个简单的 echarts 日历热力图的代码示例:
```javascript
// 引入 echarts
import echarts from 'echarts';
// 初始化 echarts 实例
const myChart = echarts.init(document.getElementById('myChart'));
// 配置 option
const option = {
// 设置图表类型为日历热力图
type: 'heatmap',
// 设置 x 轴为时间轴
calendar: {
orient: 'vertical',
yearLabel: {
show: false,
textStyle: {
color: '#333',
fontSize: 12,
},
},
monthLabel: {
show: true,
textStyle: {
color: '#333',
fontSize: 12,
},
},
dayLabel: {
show: true,
textStyle: {
color: '#333',
fontSize: 12,
},
},
range: ['2021-01-01', '2021-12-31'],
cellSize: [30, 30],
},
// 设置颜色映射规则
visualMap: {
min: 0,
max: 100,
type: 'piecewise',
orient: 'horizontal',
left: 'center',
top: '10%',
pieces: [
{ min: 0, max: 20, label: '0-20', color: '#ebedf0' },
{ min: 20, max: 40, label: '20-40', color: '#c6e48b' },
{ min: 40, max: 60, label: '40-60', color: '#7bc96f' },
{ min: 60, max: 80, label: '60-80', color: '#239a3b' },
{ min: 80, max: 100, label: '80-100', color: '#196127' },
],
},
// 设置数据
series: [
{
data: [
{ name: '2021-01-01', value: 50 },
{ name: '2021-01-02', value: 80 },
{ name: '2021-01-03', value: 20 },
// ...更多数据
],
type: 'heatmap',
},
],
};
// 使用刚指定的配置项和数据显示图表
myChart.setOption(option);
```
以上代码中,我们首先引入了 echarts 库,并初始化了一个 echarts 实例。然后,通过配置 option 对象来设置日历热力图的各种属性,包括数据、日期范围、颜色映射规则等。最后,使用 `setOption` 方法将配置项和数据显示在页面上。
当然,这只是一个简单的示例,实际的 echarts 日历热力图可能会更加复杂,需要根据具体需求做出相应的调整。
vue3 echarts 日历热力图
以下是使用Vue3和Echarts实现日历热力图的步骤:
1. 安装Echarts和Vue-Echarts
```bash
npm install echarts vue-echarts@5.0.0-beta.5
```
2. 在Vue组件中引入Echarts
```vue
<template>
<div class="calendar"></div>
</template>
<script>
import * as echarts from 'echarts';
import { use } from 'echarts/core';
import { CalendarChart } from 'echarts/charts';
import { CanvasRenderer } from 'echarts/renderers';
use([CalendarChart, CanvasRenderer]);
export default {
mounted() {
const chartDom = this.$el;
const myChart = echarts.init(chartDom);
const option = {
// 配置项
};
myChart.setOption(option);
},
};
</script>
```
3. 配置Echarts的日历热力图
```vue
<template>
<div class="calendar"></div>
</template>
<script>
import * as echarts from 'echarts';
import { use } from 'echarts/core';
import { CalendarChart } from 'echarts/charts';
import { CanvasRenderer } from 'echarts/renderers';
use([CalendarChart, CanvasRenderer]);
export default {
mounted() {
const chartDom = this.$el;
const myChart = echarts.init(chartDom);
const option = {
tooltip: {
formatter: (params) => {
return `${params.value[0]}<br>${params.value[1]}: ${params.value[2]}`;
},
}, visualMap: {
min: 0,
max: 100,
type: 'piecewise',
orient: 'horizontal',
left: 'center',
bottom: '5%',
inRange: {
color: ['#ebedf0', '#c6e48b', '#7bc96f', '#239a3b', '#196127'],
},
pieces: [
{ min: 80, label: '>=80', color: '#196127' },
{ min: 60, max: 79, label: '60-79', color: '#239a3b' },
{ min: 40, max: 59, label: '40-59', color: '#7bc96f' },
{ min: 20, max: 39, label: '20-39', color: '#c6e48b' },
{ min: 0, max: 19, label: '0-19', color: '#ebedf0' },
],
},
calendar: {
range: ['2022-01-01', '2022-12-31'],
cellSize: ['auto', 13],
top: 'middle',
left: 'center',
right: null,
bottom: null,
orient: 'vertical',
splitLine: {
show: true,
lineStyle: {
color: '#000',
width: 0.5,
type: 'solid',
},
},
itemStyle: {
color: '#fff',
borderWidth: 0.5,
borderColor: '#000',
},
yearLabel: {
show: false,
},
},
series: [
{
type: 'heatmap',
coordinateSystem: 'calendar',
data: [
['2022-01-01', 10],
['2022-01-02', 20],
// ...
['2022-12-31', 90],
],
},
],
};
myChart.setOption(option);
},
};
</script>
```
4. 在Vue组件中使用日历热力图
```vue
<template>
<div>
<CalendarHeatmap />
</div>
</template>
<script>
import CalendarHeatmap from './CalendarHeatmap.vue';
export default {
components: {
CalendarHeatmap,
},
};
</script>
```
相关推荐
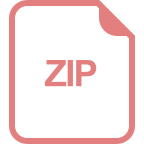
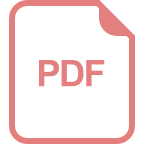












