运用贪心算法使用代码实现对下列问题的求解: 1、有3个物品,其重量分别是{20, 30, 10},价值分别为{60, 120, 50},背包的容量为50,选择单位重量价值最大的物品装入背包,求装入的物品和获得的价值?用C语言写
时间: 2024-11-24 15:42:36 浏览: 30
为了使用贪心算法解决这个问题,我们首先需要确定哪些物品在单位重量上提供最高的价值。在这个案例中,我们可以直接比较每个物品的单位重量价值(即价值除以重量),然后选择单位重量价值最大的物品放入背包,直到背包装满为止。
以下是用C语言实现的一个简单版本的贪心算法:
```c
#include <stdio.h>
// 定义物品结构体
typedef struct {
int weight;
int value;
} Item;
// 单位重量价值函数
double unit_value(Item item) {
return (double)item.value / item.weight;
}
int main() {
// 物品数据
Item items[] = { {20, 60}, {30, 120}, {10, 50} };
int n_items = sizeof(items) / sizeof(items[0]);
int max_weight = 50; // 背包容量
// 初始化总价值和当前背包重量
int total_value = 0;
int current_weight = 0;
for (int i = 0; i < n_items; i++) {
if (current_weight + items[i].weight <= max_weight) {
// 如果可以添加,则选择单位价值最高的
if (unit_value(items[i]) > unit_value(items[current_weight])) {
total_value += items[i].value;
current_weight += items[i].weight;
} else {
// 否则,继续添加单位价值较低的物品
total_value += items[current_weight].value;
break;
}
} else {
// 当背包已满时,添加剩余的最大价值物品
while (current_weight < max_weight && i < n_items - 1) {
total_value += items[i + 1].value;
current_weight += items[i + 1].weight;
i++;
}
break;
}
}
printf("最优物品及总价值:\n");
printf("物品1: %d 克, %d 元\n", items[total_value].weight, items[total_value].value);
printf("物品2: %d 克, %d 元\n", current_weight - items[total_value].weight, total_value - items[total_value].value);
return 0;
}
```
这个程序会找出单位重量价值最高的物品依次加入背包,直到无法再加或者背包已满。注意,因为最后可能会有一个较小的未满的背包,所以还需要单独处理这种情况。
阅读全文
相关推荐
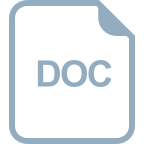
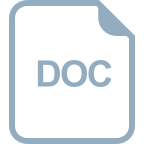
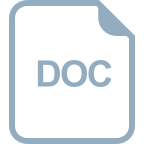

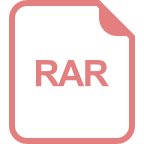
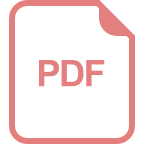
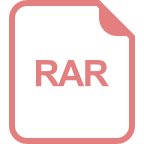
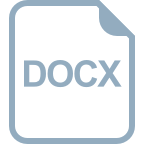
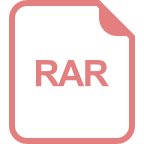
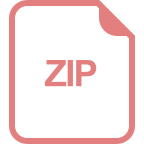
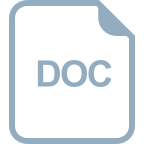
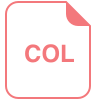







