Ubuntu使用c语言实现检测本地网卡是否存在
时间: 2024-09-13 12:15:19 浏览: 65
Ubuntu系统中,你可以通过C语言利用Linux提供的库函数来检测本地网卡的存在。一种常用的方式是使用libudev库,它提供了一种统一的方式来处理设备事件,包括网络适配器。以下是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <sys/ioctl.h>
#include <net/if.h>
#include <linux/unistd.h>
#include <linux/udev.h>
int main() {
udev_device *device;
const char *bus_name = "pci";
int ret;
if (udev_init() == -1) {
perror("udev_init failed");
return 1;
}
device = udev_new();
if (device == NULL) {
perror("udev_new failed");
return 1;
}
// 设置设备的类别过滤器
if (udev_setenv(device, "SUBSYSTEM", "net") < 0) {
perror("Setting SUBSYSTEM env var failed");
goto error;
}
if (udev_setenv(device, "DEVNAME", "*:*") < 0) { // 匹配所有网卡
perror("Setting DEVNAME env var failed");
goto error;
}
// 获取所有匹配的设备
if (udev_enumerate_add_match_subsystem(device, bus_name) < 0) {
perror("Adding match subsystem failed");
goto error;
}
if (udev_enumerate_scan(device) < 0) {
perror("Scanning devices failed");
goto error;
}
// 遍历找到的所有网卡
while ((ret = udev_enumerate_get_list_entry(device, &list_entry)) >= 0) {
udev_device *dev;
dev = list_entry.device;
const char *name = udev_device_get_devnode(dev);
printf("Found network device: %s\n", name);
}
error:
udev_unref(device);
udev_cleanup();
return ret != 0 ? 1 : 0;
}
```
这个程序首先初始化`udev`系统,设置设备过滤条件,然后扫描设备并打印出找到的网卡名称。如果在执行过程中遇到错误,会捕获异常并退出。
阅读全文
相关推荐
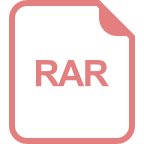
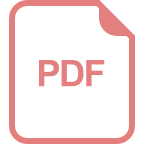
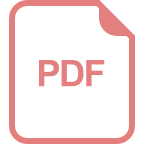















