自行选择任意的一个网站,爬取该网页上所有的图片和文字信息
时间: 2024-11-04 14:15:31 浏览: 7
爬取网页上的图片和文本信息通常涉及网络抓取技术,特别是使用Python库如BeautifulSoup和Scrapy。以一个简单的示例来说,如果你选择了`https://www.example.com`这个网站:
1. 首先,你需要安装必要的库,如requests用于发送HTTP请求获取HTML内容,然后可能是BeautifulSoup帮助解析HTML,以及例如Pillow或requests-toolbelt来下载图片。
```bash
pip install requests beautifulsoup4 pillow requests-toolbelt
```
2. 使用Python编写脚本,首先获取网页源代码:
```python
import requests
from bs4 import BeautifulSoup
url = "https://www.example.com"
response = requests.get(url)
html_content = response.text
```
3. 解析HTML内容找到所有图片链接:
```python
soup = BeautifulSoup(html_content, 'html.parser')
img_tags = soup.find_all('img')
image_links = [img['src'] for img in img_tags]
```
4. 下载图片到本地:
```python
import os
if not os.path.exists("images"):
os.makedirs("images")
for link in image_links:
try:
img_response = requests.get(link, stream=True)
with open(f"images/{link.split('/')[-1]}", 'wb') as f:
for chunk in img_response.iter_content(1024):
f.write(chunk)
except Exception as e:
print(f"Failed to download {link}: {e}")
```
5. 对于提取文本信息,可以查找并处理`<p>`、`<h1>`等标签内的文本:
```python
text_content = soup.get_text()
# 分段处理文本,去除无关字符
cleaned_text = ' '.join(text_content.split())
print(cleaned_text)
```
注意:在实际操作中,一定要遵守网站的robots.txt规则,并尊重版权,切勿进行大规模无授权的数据抓取。同时,一些网站可能会有反爬虫机制,需要适当设置延迟或使用代理IP等手段来避免被封禁。
阅读全文
相关推荐
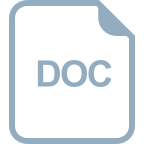
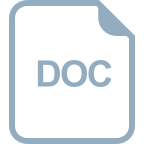
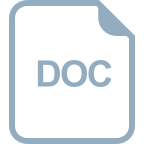
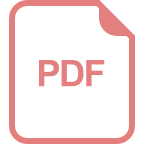
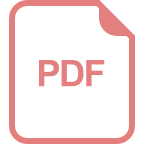
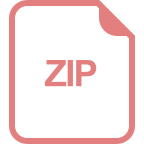
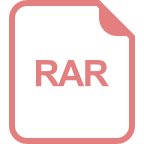
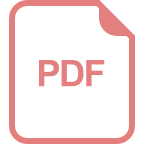
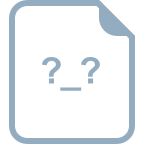
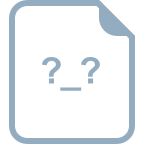
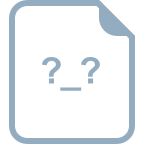
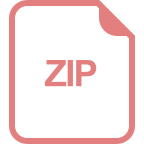
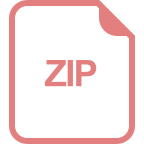
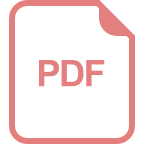
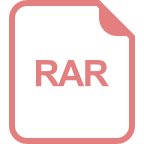