用C++语言设计代码回文是指正读、反读均相同的字符序列,如“abba”和“abdba”均是回文"good"不是回文。试设计算法判定给定的字符序列是否为回文。(提示:将一半字符人栈。)
时间: 2024-10-25 14:08:18 浏览: 10
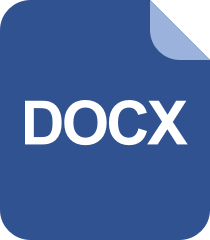
回文数是指正读和反读都相同的数.docx
在C++中,我们可以设计一个简单的算法来判断一个字符串是否为回文。这个算法的基本思路是把字符串的一半字符入栈,然后逐个比较栈顶元素和剩余未比较的字符,如果全部匹配则说明是回文。以下是详细的步骤:
```cpp
#include <iostream>
#include <stack>
#include <string>
bool isPalindrome(const std::string& str) {
// 创建一个空栈
std::stack<char> stack;
// 遍历字符串的前一半并压入栈中
for (size_t i = 0; i < str.length() / 2; ++i) {
stack.push(str[i]);
}
// 如果字符串长度是奇数,中间的字符不需要入栈直接比较
if (str.length() % 2 == 1) {
++i;
}
// 比较栈顶元素和剩余未比较的字符
for (; i < str.length(); ++i) {
if (stack.top() != str[i]) {
return false;
}
stack.pop();
}
// 如果所有字符都匹配,则返回true
return true;
}
int main() {
std::string test_strs[] = {"abba", "good", "aba"};
for (const auto& str : test_strs) {
bool result = isPalindrome(str);
if (result) {
std::cout << str << " is a palindrome.\n";
} else {
std::cout << str << " is not a palindrome.\n";
}
}
return 0;
}
```
阅读全文
相关推荐
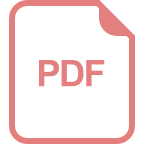















