回文是指正读反读均相同的字符序列,如ABBA,abdba均是回文,good不是回文。利用栈结构设计算法判定给定的字符串是否为回文.使用c语言或者c++完成这段代码
时间: 2024-10-07 13:08:21 浏览: 34
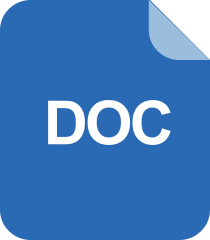
判断字符序列是否是回文

在C语言或C++中,可以使用栈的数据结构来判断一个字符串是否为回文。栈是一种后进先出(LIFO)的数据结构,非常适合用于处理这个问题。下面是使用C++编写的代码示例:
```cpp
#include <iostream>
#include <stack>
#include <string>
bool isPalindrome(std::string str) {
std::stack<char> stack;
// 将字符串的前半部分压入栈中
for (int i = 0; i < str.size() / 2; ++i) {
stack.push(str[i]);
}
// 检查剩余的部分(如果长度为奇数,则忽略中间的字符)
if (str.size() % 2 == 1) {
--i;
}
// 反转栈顶元素,比较与当前未检查位置的字符
while (!stack.empty()) {
if (str[i] != stack.top()) {
return false;
}
stack.pop();
++i;
}
return true;
}
int main() {
std::string input;
std::cout << "Enter a string: ";
std::cin >> input;
if (isPalindrome(input)) {
std::cout << input << " is a palindrome." << std::endl;
} else {
std::cout << input << " is not a palindrome." << std::endl;
}
return 0;
}
```
这个程序首先将字符串的一半字符放入栈中,然后逐个从字符串的另一端开始取出并对比栈顶元素。如果在任何时候字符不匹配,就返回false;全部匹配则返回true。
阅读全文
相关推荐
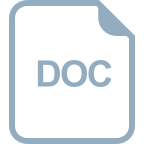
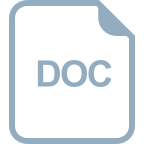













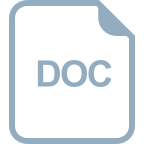
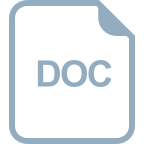