springboot batis mysql5.7
时间: 2023-09-24 12:01:18 浏览: 189
Spring Boot是一个基于Java的开源框架,用于简化Spring应用程序的开发和部署。它提供了许多开箱即用的特性,例如自动配置、内嵌式容器等,使得开发者可以更加便捷地进行应用程序的构建和部署。
MySql是一种常用的关系型数据库管理系统,而5.7则是其中的一个版本。它在性能、安全性和稳定性上有一定的优势,能够满足大多数应用场景的需求。与Spring Boot结合使用时,可以利用Spring Boot的自动配置功能轻松地与MySql进行集成。
而MyBatis是一个持久层框架,用于简化数据库访问的编码工作。它与Spring Boot和MySql的结合可以更进一步地简化开发流程。与传统的JDBC相比,MyBatis提供了更加简洁的API,能够更易于维护和修改SQL语句。同时,MyBatis还提供了一些高级特性,例如缓存、动态SQL等,能够进一步提升应用程序的性能和灵活性。
因此,使用Spring Boot、MyBatis和MySql 5.7的组合,可以极大地提高开发效率和应用程序的性能。开发者只需要简单地配置一些基本参数,即可快速搭建一个具备数据库持久化功能的应用程序。同时,Spring Boot还提供了一些监控和调试工具,使开发者能够更方便地进行应用程序的排错和性能优化。综上所述,Spring Boot、MyBatis和MySql 5.7是一种非常强大的开发组合,可以帮助开发者快速构建高性能、可维护的应用程序。
相关问题
spring boot + sping mvc + jpa/batis + mysql + jwt都是干嘛的
这些技术都是用于构建现代化的Web应用程序的。具体来说:
- Spring Boot:是一个基于Spring框架的开发框架,可以快速地创建独立的、基于Spring的应用程序,并且提供了很多开箱即用的功能,如自动配置、内嵌Tomcat服务器等。
- Spring MVC:是一个基于Java的Web框架,用于构建Web应用程序。Spring MVC提供了一种MVC(模型-视图-控制器)模式,可以使开发人员将应用程序的不同方面分离开来,以便更好地维护和扩展应用程序。
- JPA/Batis:JPA(Java Persistence API)是Java EE平台的一个ORM(对象关系映射)标准,可以方便地将Java对象映射到关系数据库中。Batis是一个Java持久层框架,提供了一种将Java对象映射到SQL语句的方式。
- MySQL:是一个开源的关系型数据库管理系统,广泛应用于Web应用程序中。
- JWT(JSON Web Token):是一种开放标准,用于在不同系统之间传输信息。JWT通常用于身份验证和授权,可以帮助开发人员构建安全的Web应用程序。
implement the shipping REST APIs with spring boot + sping mvc + jpa/batis + mysql + jwt
Sure, here's an outline of how you can implement the shipping REST APIs using Spring Boot, Spring MVC, JPA/Batis, MySQL, and JWT:
1. Set up a Spring Boot project with the necessary dependencies for Spring MVC, JPA/Batis, MySQL, and JWT. You can use Spring Initializr to generate the project structure and add the dependencies.
2. Define the domain model for the shipping application, including entities such as Order, Product, Customer, and ShippingAddress. Map the entities to database tables using JPA annotations or MyBatis mapper XML files.
3. Implement the repository layer to perform CRUD operations on the database using JPA or MyBatis. You can use Spring Data JPA or MyBatis-Spring to simplify the implementation.
4. Define the REST API endpoints for the shipping application using Spring MVC annotations. Use JWT for authentication and authorization of the API endpoints.
5. Implement the service layer to perform business logic operations such as calculating shipping costs, validating orders, and processing payments. Use dependency injection to inject the repository and other services into the service layer.
6. Write unit tests to ensure that the application logic is working correctly. You can use JUnit and Mockito to write the tests.
7. Deploy the application to a server and test the API endpoints using a tool such as Postman.
Here's some example code to get you started:
```java
@RestController
@RequestMapping("/api/orders")
public class OrderController {
@Autowired
private OrderService orderService;
@PostMapping("/")
public ResponseEntity<Order> createOrder(@RequestBody Order order) {
Order createdOrder = orderService.createOrder(order);
return ResponseEntity.ok(createdOrder);
}
@GetMapping("/{id}")
public ResponseEntity<Order> getOrder(@PathVariable("id") Long orderId) {
Order order = orderService.getOrder(orderId);
return ResponseEntity.ok(order);
}
// Other API endpoints for updating and deleting orders
}
@Service
public class OrderService {
@Autowired
private OrderRepository orderRepository;
public Order createOrder(Order order) {
// Calculate shipping costs and validate the order
order.setShippingCosts(10.0);
order.setTotalPrice(order.getProducts().stream()
.mapToDouble(Product::getPrice)
.sum() + order.getShippingCosts());
return orderRepository.save(order);
}
public Order getOrder(Long orderId) {
return orderRepository.findById(orderId)
.orElseThrow(() -> new NotFoundException("Order not found"));
}
// Other service methods for updating and deleting orders
}
@Repository
public interface OrderRepository extends JpaRepository<Order, Long> {
}
```
This code defines a REST API endpoint for creating orders and getting orders by ID. The order creation logic is implemented in the OrderService class, which calculates shipping costs and saves the order to the database using the OrderRepository interface. JWT authentication and authorization can be added to the API endpoints using Spring Security.
阅读全文
相关推荐
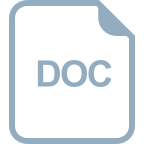
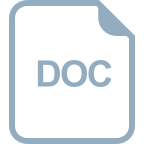
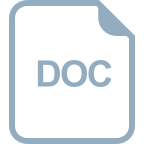
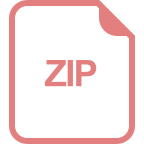
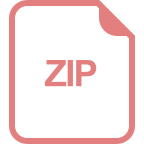
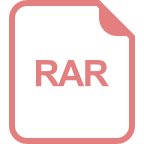
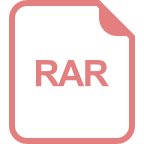
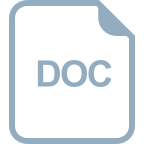
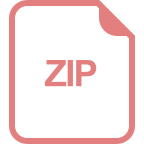
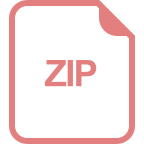
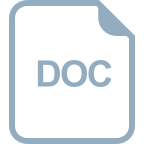
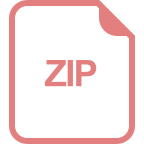
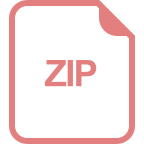



