使用keras做多维多变量多步时间预测
时间: 2023-06-24 11:08:40 浏览: 53
这里提供一个使用Keras实现多维多变量多步时间预测的示例。假设我们有一个包含多个特征的时间序列数据集,我们需要预测未来n个时间步长的数据。
首先,我们需要将数据集转换为适合Keras模型的格式。我们可以通过将时间序列划分为输入和输出序列来实现。例如,如果我们要预测未来3个时间步长的数据,我们可以使用前面的12个时间步长作为输入,第13到15时间步长作为输出。下面是一个将数据集转换为输入输出序列的函数:
```python
import numpy as np
def split_sequence(sequence, n_steps_in, n_steps_out):
X, y = [], []
for i in range(len(sequence)):
end_ix = i + n_steps_in
out_end_ix = end_ix + n_steps_out-1
if out_end_ix > len(sequence):
break
seq_x, seq_y = sequence[i:end_ix, :], sequence[end_ix-1:out_end_ix, :]
X.append(seq_x)
y.append(seq_y)
return np.array(X), np.array(y)
```
接下来,我们需要定义Keras模型。我们可以使用多个层来构建一个序列模型,例如LSTM和Dense层。下面是一个简单的模型,包含两个LSTM层和一个Dense层:
```python
from keras.models import Sequential
from keras.layers import LSTM, Dense
n_features = X_train.shape[2]
n_steps_in, n_steps_out = 12, 3
model = Sequential()
model.add(LSTM(100, activation='relu', input_shape=(n_steps_in, n_features)))
model.add(LSTM(100, activation='relu'))
model.add(Dense(n_steps_out))
model.compile(optimizer='adam', loss='mse')
```
接下来,我们可以训练模型并使用它来预测未来的时间步长。下面是一个训练和预测模型的示例:
```python
# 训练模型
model.fit(X_train, y_train, epochs=100)
# 预测未来3个时间步长的数据
x_input = X_test[0]
x_input = x_input.reshape((1, n_steps_in, n_features))
yhat = model.predict(x_input, verbose=0)
print(yhat)
```
需要注意的是,我们需要将预测的输出重新调整为原始数据集的规模,以便进行比较和可视化。
相关推荐
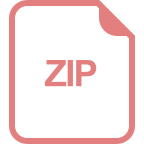
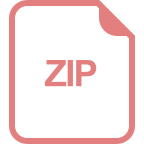
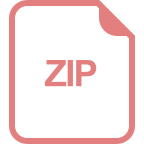














