lru页面置换算法python
时间: 2024-11-19 16:14:56 浏览: 16
LRU (Least Recently Used) 页面置换算法是一种常用的计算机内存管理策略,主要用于解决虚拟内存中的缓存替换问题。当系统内存不足时,它会淘汰最近最少使用的页面,以便腾出空间给新访问的数据。
在Python中,虽然标准库并未直接提供对LRU算法的实现,但我们可以通过第三方库如`cachetools`来轻松地应用这个概念。`cachetools.lru`模块提供了LRU Cache的功能,例如:
```python
from cachetools import LRUCache
# 创建一个大小为10的LRU缓存
cache = LRUCache(maxsize=10)
# 存储数据
cache['key1'] = 'value1'
cache['key2'] = 'value2'
# 如果缓存已满,最近最少使用的键会被自动移除,比如 'key3' 被替换掉
cache['key3'] = 'value3'
# 获取值
print(cache.get('key1')) # 输出 'value1'
```
当你尝试获取一个不存在于缓存中的键时,`get()`函数会返回None。这就是LRU缓存的工作原理。
相关问题
LRU页面置换算法代码
以下是LRU页面置换算法的Python代码实现:
```python
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
self.keys = []
def get(self, key):
if key in self.cache:
self.keys.remove(key)
self.keys.append(key)
return self.cache[key]
return -1
def put(self, key, value):
if key in self.cache:
self.keys.remove(key)
elif len(self.cache) == self.capacity:
del self.cache[self.keys[0]]
del self.keys[0]
self.keys.append(key)
self.cache[key] = value
```
在这个实现中,我们使用了一个字典来缓存页面和它们的值,并使用一个列表来跟踪页面的访问顺序。当一个页面被访问时,我们将它移动到列表的末尾。当缓存满时,我们从字典中删除列表的第一个页面并从列表中删除,以便为新页面腾出空间。
LRU页面置换算法实现
LRU页面置换算法实现需要以下步骤:
1. 建立一个双向链表,用于维护页面的使用顺序。链表头表示最近最少使用的页面,链表尾表示最近使用的页面。
2. 建立一个哈希表,用于快速查找页面是否在内存中,并保存页面在链表中的位置。
3. 当需要访问一个页面时,先在哈希表中查找是否在内存中。如果在内存中,将该页面移动到链表头;如果不在内存中,进行页面置换。
4. 当内存不足时,需要选择一个页面进行置换。选择链表尾的页面进行置换,因为它是最近最少使用的页面。
5. 从链表尾删除该页面,并从哈希表中删除该页面的记录。
6. 将新页面加入链表头,并在哈希表中记录该页面的位置。
7. 重复上述步骤,直到访问完所有页面。
下面是一个简单的Python实现:
```python
class LRUCache:
def __init__(self, capacity: int):
self.capacity = capacity
self.cache = {}
self.head = Node(0, 0)
self.tail = Node(0, 0)
self.head.next = self.tail
self.tail.prev = self.head
def get(self, key: int) -> int:
if key in self.cache:
node = self.cache[key]
self._remove(node)
self._add(node)
return node.val
else:
return -1
def put(self, key: int, value: int) -> None:
if key in self.cache:
self._remove(self.cache[key])
node = Node(key, value)
self.cache[key] = node
self._add(node)
if len(self.cache) > self.capacity:
node = self.head.next
self._remove(node)
del self.cache[node.key]
def _add(self, node):
p = self.tail.prev
p.next = node
node.prev = p
node.next = self.tail
self.tail.prev = node
def _remove(self, node):
p = node.prev
n = node.next
p.next = n
n.prev = p
class Node:
def __init__(self, k, v):
self.key = k
self.val = v
self.prev = None
self.next = None
```
其中,LRUCache类实现了LRU页面置换算法,get方法用于获取页面,put方法用于添加页面,_add和_remove方法用于链表操作,Node类表示链表节点。
阅读全文
相关推荐
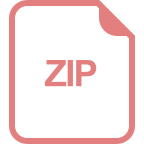
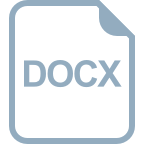
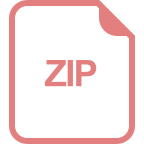
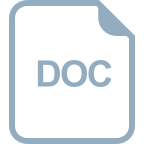




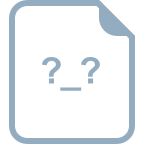
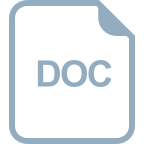






