使用python代码,实现指纹图像的增强,指纹纹线的细化,根据细化指纹图像提取端点和交叉点,在原始图像中标记并输出坐标
时间: 2023-12-14 22:04:53 浏览: 50
以下是使用Python和OpenCV库实现指纹图像增强、细化以及特征点提取的代码。请确保已经安装了OpenCV库。
```python
import cv2
# 读取指纹图像
img = cv2.imread('fingerprint.png', 0)
# 增强图像对比度
clahe = cv2.createCLAHE(clipLimit=2.0, tileGridSize=(8,8))
enhanced_img = clahe.apply(img)
# 二值化图像
_, bin_img = cv2.threshold(enhanced_img, 0, 255, cv2.THRESH_BINARY+cv2.THRESH_OTSU)
# 细化图像
thinned_img = cv2.ximgproc.thinning(bin_img)
# 提取端点和交叉点
def get_feature_points(img):
endpoints = cv2.ximgproc.getFastFeatureDetector_create(threshold=10, nonmaxSuppression=True)
keypoints1 = endpoints.detect(img)
crosspoints = cv2.ximgproc.getFastFeatureDetector_create(threshold=10, nonmaxSuppression=False)
keypoints2 = crosspoints.detect(img)
return keypoints1, keypoints2
endpoints, crosspoints = get_feature_points(thinned_img)
# 在原始图像中标记特征点并输出坐标
for point in endpoints:
cv2.circle(img, (int(point.pt[0]), int(point.pt[1])), 5, (0, 0, 255), -1)
print("Endpoint: ({}, {})".format(int(point.pt[0]), int(point.pt[1])))
for point in crosspoints:
cv2.circle(img, (int(point.pt[0]), int(point.pt[1])), 5, (255, 0, 0), -1)
print("Crosspoint: ({}, {})".format(int(point.pt[0]), int(point.pt[1])))
# 显示原始图像和标记后的特征点图像
cv2.imshow("Original Image", img)
cv2.imshow("Thinned Image", thinned_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要将代码中的 `fingerprint.png` 替换为实际指纹图像的文件路径。执行代码后,将会显示原始图像和细化后的图像,并在原始图像中标记出端点和交叉点,并输出它们的坐标。
相关推荐
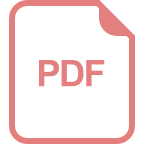
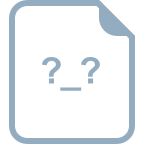
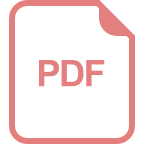
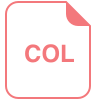
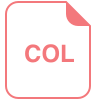












