verilog例化例子
时间: 2024-09-23 18:11:15 浏览: 33
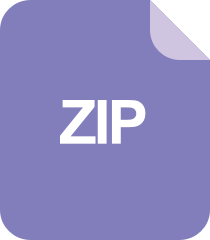
verilog自动例化程序及例子
Verilog是一种硬件描述语言,用于设计和验证数字电路系统。在Verilog中,例化(Instantiation)是指创建模块实例的过程,这使得一个模块可以在更大的系统结构中复用。下面是一个简单的Verilog模块和其例化的基本示例:
```verilog
// 定义一个名为"Adder"的模块,它有输入端a、b和输出端sum
module Adder (
input wire [7:0] a,
input wire [7:0] b,
output reg [7:0] sum
);
// 模块内部实现略...
assign sum = a + b;
endmodule
// 现在我们例化这个Adder模块
module top;
// 通过使用"."运算符和模块名来实例化Adder模块
wire [7:0] result;
Adder add1 (.a(8'b123), .b(8'b456), .sum(result)); // 给输入提供数据并声明结果变量
// 其他电路连接...
endmodule
```
在这个例子中,`top`模块中有一个`add1`实例,它是`Adder`模块的一个副本。`add1`的输入端`a`和`b`被赋予具体的值,并将`sum`的结果保存到`result`信号上。
阅读全文
相关推荐
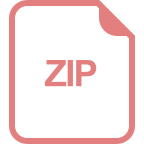
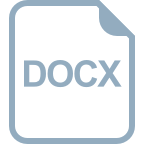

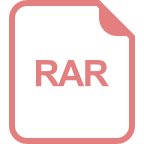
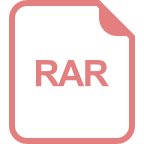
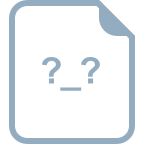











