二叉树的层序遍历链表实现
时间: 2024-05-21 10:09:51 浏览: 19
二叉树的层序遍历链表实现是指将二叉树按照层次遍历,并将同一层的节点放在同一个链表中。实现过程中需要借助队列,具体实现步骤如下:
1. 首先将根节点入队;
2. 然后进入循环,当队列非空时,依次将队首元素弹出,并将其值存入当前链表中;
3. 若该节点存在左子节点,则将左子节点入队;
4. 若该节点存在右子节点,则将右子节点入队;
5. 循环直至队列为空,此时遍历完成。
以下是二叉树层序遍历链表实现的C++代码示例:
```c++
#include <iostream>
#include <queue>
using namespace std;
struct TreeNode {
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
};
struct ListNode {
int val;
ListNode* next;
ListNode(int x) : val(x), next(NULL) {}
};
vector<ListNode*> levelOrder(TreeNode* root) {
vector<ListNode*> res;
if (!root) return res; // 如果根节点为空,返回空vector
queue<TreeNode*> q;
q.push(root);
while (!q.empty()) {
int n = q.size();
ListNode* head = nullptr;
ListNode* tail = nullptr;
for (int i = 0; i < n; ++i) {
TreeNode* node = q.front();
q.pop();
if (node->left) q.push(node->left);
if (node->right) q.push(node->right);
if (head == nullptr) {
head = tail = new ListNode(node->val);
} else {
tail->next = new ListNode(node->val);
tail = tail->next;
}
}
res.push_back(head);
}
return res;
}
```
相关推荐
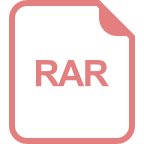














