function [IDX, isnoise]=PlotClusterinResult(X,epsilon,MinPts) C=0; n=size(X,1); IDX=zeros(n,1); % 初始化全部为0,即全部为噪音点 D=pdist2(X,X); visited=false(n,1); isnoise=false(n,1); for i=1:n if ~visited(i) visited(i)=true; Neighbors=RegionQuery(i); if numel(Neighbors)<MinPts % X(i,:) is NOISE isnoise(i)=true; else C=C+1; ExpandCluster(i,Neighbors,C); end end end function ExpandCluster(i,Neighbors,C) IDX(i)=C; k = 1; while true j = Neighbors(k); if ~visited(j) visited(j)=true; Neighbors2=RegionQuery(j); if numel(Neighbors2)>=MinPts Neighbors=[Neighbors Neighbors2]; %#ok end end if IDX(j)==0 IDX(j)=C; end k = k + 1; if k > numel(Neighbors) break; end end end function Neighbors=RegionQuery(i) Neighbors=find(D(i,:)<=epsilon); end end
时间: 2024-02-10 08:19:22 浏览: 74
从代码中看,你定义了一个名为 "PlotClusterinResult" 的函数,但是这个函数并没有返回任何值,而是定义了三个输入参数 "X"、"epsilon" 和 "MinPts"。这个函数内部还定义了另外三个函数 "ExpandCluster"、"RegionQuery" 和 "PlotClusterinResult",它们被定义为 "PlotClusterinResult" 函数的嵌套函数。
根据你的错误提示 "PlotClusterinResult 输入参数的数目不足。",你在调用这个函数时没有给它提供足够的输入参数。你需要检查一下调用 "PlotClusterinResult" 函数的代码,确保你给它提供了正确数量的输入参数,并且参数的类型和顺序都正确。
在这个函数中,使用了一个变量 "MinPts",但是它在函数声明中没有被定义,你需要在函数声明中加上 "MinPts" 的定义,以便正确传递这个参数。
另外,这个函数似乎是实现了基于密度的聚类算法 DBSCAN,如果你想要使用这个函数进行聚类,你还需要将数据集 "X"、半径 "epsilon" 和最小点数 "MinPts" 作为输入参数传递给 "PlotClusterinResult" 函数。
相关问题
Runs MNIST training with differential privacy. """ Using matrix project to compress the gradient matrix """ def compress(grad, num_k, power_iter=1): return B, G_hat """ Complete the function of per-example clip """ def clip_column(tsr, clip_value=1.0): return def train(args, model, device, train_loader, optimizer, epoch, loss_func, clip_value): model.train() # criterion = nn.CrossEntropyLoss() losses = [] for _batch_idx, (data, target) in enumerate(tqdm(train_loader)): data, target = data.to(device), target.to(device) batch_grad_list = [] optimizer.zero_grad() output = model(data) loss = loss_func(output, target) if not args.disable_dp: with backpack(BatchGrad()): loss.backward() for p in model.parameters(): batch_grad_list.append(p.grad_batch.reshape(p.grad_batch.shape[0], -1)) #compose gradient into Matrix del p.grad_batch """ Using project method to compress the gradient """ if args.using_compress: #per-example clip else: """ Complete the code of DPSGD """ else: loss.backward() try: for p in model.parameters(): del p.grad_batch except: pass optimizer.step() losses.append(loss.item()) #get the num of the training dataset from train_loader if not args.disable_dp: epsilon = get_epsilon(epoch, delta=args.delta, sigma=args.sigma, sensitivity=clip_value, batch_size=args.batch_size, training_nums=len(train_loader)*args.batch_size) print( f"Train Epoch: {epoch} \t" f"Loss: {np.mean(losses):.6f} " f"(ε = {epsilon:.2f}, δ = {args.delta})" ) else: print(f"Train Epoch: {epoch} \t Loss: {np.mean(losses):.6f}")
这段代码是运行带有差分隐私的MNIST训练,其中使用矩阵项目压缩梯度矩阵。函数compress(grad, num_k, power_iter=1)用于压缩梯度矩阵,该函数返回B和G_hat。函数clip_column(tsr, clip_value=1.0)是用于每个样本的剪切函数,需要你完成代码。train函数是训练函数,其中loss_func是损失函数,clip_value是剪切值,如果不禁用差分隐私,则会计算epsilon值并打印。
matlab6.2:函数 ,求: a. 绘制[0 ,8]区间内的图形,加注x,y轴及图形名称; b. 最大值和最小值; c. 零值;
在MATLAB 6.2中,你可以使用`fplot`函数来绘制函数图形,并结合其他命令添加坐标轴、标题以及查找极值和零点。这里是一个基本步骤的例子:
**a. 绘制并标注图形**
```matlab
% 定义需要绘制的函数,例如y = x^2 + 1 (这是一个简单的二次函数)
function y = func(x)
y = x.^2 + 1;
end
% 创建x值范围
x = linspace(0, 8); % [0, 8]区间的等间距数值
% 使用fplot绘制函数
figure; % 创建新窗口
fplot(func, [0 8]); % 绘图,注意第二个参数是绘图区域
% 添加坐标轴和标题
xlabel('X轴'); % x轴标签
ylabel('Y轴'); % y轴标签
title('函数图像 - 示例'); % 图形标题
```
**b. 查找最大值和最小值**
```matlab
% 找到最大值
[max_val, max_idx] = max(func(x));
fprintf('最大值: %f, 出现在x=%f\n', max_val, x(max_idx));
% 找到最小值
[min_val, min_idx] = min(func(x));
fprintf('最小值: %f, 出现在x=%f\n', min_val, x(min_idx));
```
**c. 查找零值**
由于给定的是一个连续函数,如果函数不是线性的,可能不存在整数解。我们可以使用`find`函数寻找近似的零点:
```matlab
% 寻找函数接近零的小误差点(例如,小于1e-5)
epsilon = 1e-5;
zero_indices = find(abs(func(x)) < epsilon);
if ~isempty(zero_indices)
fprintf('找到的零点: x = %f\n', x(zero_indices));
else
fprintf('未找到精确的零点。\n');
end
```
记得运行这些代码前先确认MATLAB 6.2环境已经设置好,因为有些现代功能可能不可用。
阅读全文
相关推荐





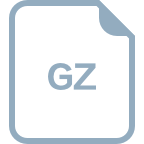





