请编写一个python程序,实现CondConv卷积的代码。
时间: 2023-12-10 10:42:58 浏览: 221
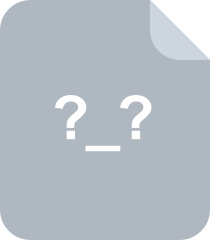
卷积python代码

CondConv(条件卷积)是一种可以根据输入数据动态调整卷积核的方法,它可以根据输入的特征图自适应地选择卷积核,从而使得模型更加灵活。以下是一个基于PyTorch实现CondConv的示例代码:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class CondConv2d(nn.Module):
def __init__(self, in_channels, out_channels, kernel_size, stride=1, padding=0, dilation=1, groups=1, bias=True):
super(CondConv2d, self).__init__()
self.in_channels = in_channels
self.out_channels = out_channels
self.kernel_size = kernel_size
self.stride = stride
self.padding = padding
self.dilation = dilation
self.groups = groups
self.bias = bias
self.weight = nn.Parameter(torch.Tensor(out_channels, in_channels // groups, kernel_size, kernel_size))
if bias:
self.bias = nn.Parameter(torch.Tensor(out_channels))
else:
self.register_parameter('bias', None)
self.cond_weight = nn.Parameter(torch.Tensor(out_channels, in_channels // groups, kernel_size, kernel_size))
self.reset_parameters()
def reset_parameters(self):
nn.init.kaiming_uniform_(self.weight, a=math.sqrt(5))
nn.init.kaiming_uniform_(self.cond_weight, a=math.sqrt(5))
if self.bias is not None:
fan_in, _ = nn.init._calculate_fan_in_and_fan_out(self.weight)
bound = 1 / math.sqrt(fan_in)
nn.init.uniform_(self.bias, -bound, bound)
def forward(self, x, cond):
weight = self.weight * self.cond_weight.expand_as(self.weight)
return F.conv2d(x, weight, self.bias, self.stride, self.padding, self.dilation, self.groups)
```
这个CondConv2d类继承自nn.Module,实现了一个基本的卷积层,除了标准的卷积参数(输入特征图通道数、输出特征图通道数、卷积核大小等)外,还包括了一个cond参数,用于传递额外的条件信息。在forward函数中,我们首先将cond参数通过一个和权重相同维度的cond_weight参数进行扩展,然后使用此扩展后的cond_weight参数和原始权重weight参数进行乘法操作,从而实现根据条件动态调整卷积核的目的。最后,我们使用F.conv2d函数进行卷积运算,并返回结果。
需要注意的是,在实现CondConv时,我们需要为每个卷积核都设立一个对应的条件参数,这将导致模型参数数量的显著增加,因此需要谨慎使用。
阅读全文
相关推荐
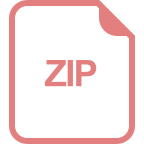
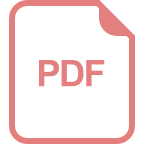
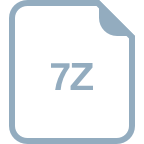
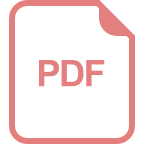
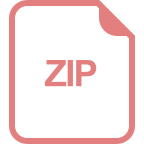
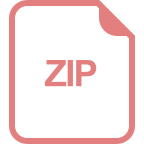
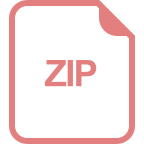
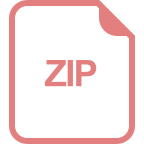
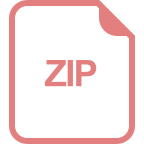
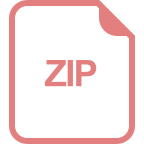
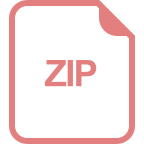
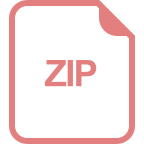
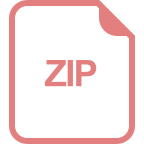