python 复杂抠图
时间: 2023-06-11 19:07:46 浏览: 53
在Python中,可以使用许多库进行图像处理和抠图,其中最常用的是OpenCV和Pillow。
使用OpenCV进行抠图的步骤如下:
1. 读取图片并转换为灰度图像
```python
import cv2
img = cv2.imread("image.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 对图像进行二值化处理,将背景变为黑色
```python
ret, thresh = cv2.threshold(gray, 0, 255, cv2.THRESH_BINARY_INV+cv2.THRESH_OTSU)
```
3. 进行形态学操作,填充目标区域,使其连通
```python
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3, 3))
closing = cv2.morphologyEx(thresh, cv2.MORPH_CLOSE, kernel, iterations=2)
```
4. 找到目标区域的轮廓,并用最小矩形框将其框起来
```python
contours, hierarchy = cv2.findContours(closing, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
rect = cv2.minAreaRect(contour)
box = cv2.boxPoints(rect)
box = np.int0(box)
cv2.drawContours(img, [box], 0, (0, 0, 255), 2)
```
5. 将目标区域外的部分涂为白色,即抠图完成
```python
mask = np.zeros_like(img)
cv2.drawContours(mask, [box], 0, (255, 255, 255), -1)
result = np.bitwise_and(img, mask)
```
使用Pillow进行抠图的步骤如下:
1. 读取图片并将其转换为RGBA模式
```python
from PIL import Image
img = Image.open("image.png")
img = img.convert("RGBA")
```
2. 获取图像中每个像素的RGBA值,并将背景像素的alpha通道值设为0
```python
width, height = img.size
for x in range(width):
for y in range(height):
r, g, b, a = img.getpixel((x, y))
if r > 200 and g > 200 and b > 200:
a = 0
img.putpixel((x, y), (r, g, b, a))
```
3. 将抠出的图像保存到文件
```python
img.save("result.png")
```
相关推荐
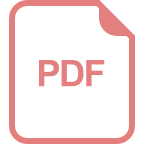









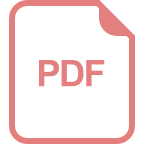
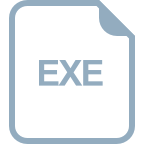
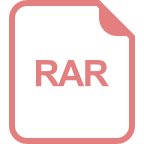