python label 闪烁
时间: 2023-10-12 11:18:27 浏览: 87
您可以使用`after()`方法在一定时间后改变标签的文本或颜色来制作闪烁效果。
下面是一个例子,它会使标签在1秒钟内交替显示“Hello”和“World”:
```python
import tkinter as tk
root = tk.Tk()
label = tk.Label(root, text="Hello", font=("Arial", 30))
label.pack()
def blink():
if label["text"] == "Hello":
label.config(text="World", fg="red")
else:
label.config(text="Hello", fg="black")
root.after(1000, blink)
blink()
root.mainloop()
```
在这个例子中,我们定义了一个`blink()`函数,它会检查标签的当前文本,然后根据需要改变它的文本和前景色。然后,我们使用`after()`方法安排`blink()`在1秒钟后再次运行,从而创建一个无限循环的闪烁效果。
相关问题
python label
在Python中,Label是Tkinter库中用来显示文本或位图的小部件。Label小部件可以用来显示简单的文本或图像,以及应用程序的其他用户界面元素。
下面是一些关于Python Label的用法的例子:
- 例子1: 创建一个具有raised属性的标签,使用relief="raised"参数来设置标签的样式为凸起效果。引用
- 例子2: 创建一个具有raised属性的标签,并设置背景色为lightyellow,使用padx和pady参数来设置标签的水平和垂直内边距。引用
- 例子3: 创建一个标签,并在其位置显示一个error位图,使用bitmap参数来设置标签的位图样式。引用
这些示例演示了如何创建不同样式和功能的标签,你可以根据自己的需求进行进一步的定制和调整。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [Python之tkinter 标签Label的基本应用](https://blog.csdn.net/qq_44168690/article/details/104560447)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
python label 刷新
要刷新 Python 中的标签,可以使用 `label.config(text="new text")` 方法,其中 `label` 是 Label 控件的名称,`text` 是要更新的文本。例如,以下代码将更新名为 `my_label` 的 Label 控件的文本为 "Hello World":
```
my_label.config(text="Hello World")
```
如果需要在特定时间间隔后刷新标签,可以使用 `after()` 方法来设置定时器。以下代码将在 5 秒后更新标签文本为 "New Text":
```
import tkinter as tk
root = tk.Tk()
my_label = tk.Label(root, text="Original Text")
my_label.pack()
def update_label():
my_label.config(text="New Text")
root.after(5000, update_label)
root.mainloop()
```
在上面的代码中,我们使用了 `after()` 方法来调用 `update_label()` 函数,在 5 秒后更新 Label 控件的文本。
相关推荐
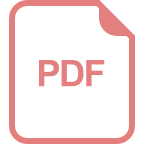












