基于Scikit-Learn利用 MNIST 数据集设计并训练神经网络模型,实现输入手写图片,输出其对应的数字。
时间: 2024-05-01 14:19:18 浏览: 9
首先,我们需要准备 MNIST 数据集。可以使用 Scikit-Learn 中的 `fetch_openml` 函数来获取该数据集:
```python
from sklearn.datasets import fetch_openml
mnist = fetch_openml('mnist_784')
```
接下来,我们需要将数据集分为训练集和测试集,并对数据进行预处理。我们可以将像素值缩放到 0 到 1 之间,并将标签转换为整数:
```python
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler, LabelEncoder
X_train, X_test, y_train, y_test = train_test_split(mnist.data, mnist.target, test_size=0.2, random_state=42)
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
encoder = LabelEncoder()
y_train = encoder.fit_transform(y_train)
y_test = encoder.transform(y_test)
```
现在,我们可以定义神经网络模型。我们使用 Keras 库来定义模型,并使用 TensorFlow 作为后端。我们选用一个包含两个隐藏层的多层感知器(MLP)模型:
```python
from keras.models import Sequential
from keras.layers import Dense
model = Sequential()
model.add(Dense(256, activation='relu', input_shape=(784,)))
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
model.compile(loss='sparse_categorical_crossentropy', optimizer='adam', metrics=['accuracy'])
```
训练模型时,我们可以使用 `fit` 函数。我们将训练集和测试集传递给该函数,并设置一些超参数,如批量大小和训练周期:
```python
history = model.fit(X_train, y_train, batch_size=64, epochs=10, validation_data=(X_test, y_test))
```
最后,我们可以使用训练好的模型对手写数字图片进行分类。我们需要将图片转换为大小为 28x28 的灰度图像,并将其展平为大小为 784 的向量。然后,我们可以使用 `predict` 函数对图片进行分类:
```python
import numpy as np
from PIL import Image
def predict_image(model, image_path):
img = Image.open(image_path).convert('L')
img = img.resize((28, 28))
img = np.array(img).reshape(1, 784)
img = scaler.transform(img)
prediction = model.predict(img)[0]
return np.argmax(prediction)
```
现在我们已经准备好了对手写数字图片进行分类。我们可以使用以下代码来测试模型:
```python
image_path = 'image.png'
prediction = predict_image(model, image_path)
print(f'The predicted digit is: {prediction}')
```
请注意,此处 `image.png` 是一个手写数字图片的示例。在使用该代码之前,请将其替换为您自己的图片。
相关推荐
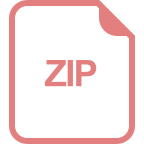
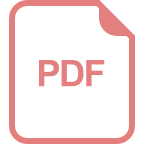
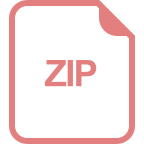














