image = image.numpy().squeeze()
时间: 2023-11-26 13:04:16 浏览: 60
这行代码的作用是将一个张量(Tensor)转换成一个NumPy数组,并通过squeeze函数去掉维数为1的维度。换句话说,如果原来的张量是一个4维张量,且其中有一个维度大小为1,那么squeeze函数将其压缩,使得这个维度消失,转换成一个3维数组。最终得到的结果是一个3维的NumPy数组,可以用于图像的显示或其他操作。
相关问题
from skimage.segmentation import slic, mark_boundaries import torchvision.transforms as transforms import numpy as np from PIL import Image import matplotlib.pyplot as plt import torch.nn as nn import torch # 定义超像素池化层 class SuperpixelPooling(nn.Module): def init(self, n_segments): super(SuperpixelPooling, self).init() self.n_segments = n_segments def forward(self, x): # 使用 SLIC 算法生成超像素标记图 segments = slic(x.numpy().transpose(1, 2, 0), n_segments=self.n_segments, compactness=10) # 将超像素标记图转换为张量 segments_tensor = torch.from_numpy(segments).unsqueeze(0).unsqueeze(0) # 将张量 x 与超像素标记图张量 segments_tensor 进行逐元素相乘 pooled = x * segments_tensor.float() # 在超像素维度上进行最大池化 pooled = nn.AdaptiveMaxPool2d((self.n_segments, 1))(pooled) # 压缩超像素维度 pooled = pooled.squeeze(3) # 返回池化后的特征图 return pooled # 加载图像 image = Image.open('3.jpg') # 转换为 PyTorch 张量 transform = transforms.ToTensor() img_tensor = transform(image).unsqueeze(0) # 将 PyTorch 张量转换为 Numpy 数组 img_np = img_tensor.numpy().transpose(0, 2, 3, 1)[0] # 使用 SLIC 算法生成超像素标记图 segments = slic(img_np, n_segments=60, compactness=10) # 将超像素标记图转换为张量 segments_tensor = torch.from_numpy(segments).unsqueeze(0).float() # 将超像素索引映射可视化 plt.imshow(segments, cmap='gray') plt.show() # 将 Numpy 数组转换为 PIL 图像 segment_img = Image.fromarray((mark_boundaries(img_np, segments) * 255).astype(np.uint8)) # 保存超像素索引映射可视化 segment_img.save('segment_map.jpg') # 使用超像素池化层进行池化 pooling_layer = SuperpixelPooling(n_segments=60) pooled_tensor = pooling_layer(img_tensor) # 将超像素池化后的特征图可视化 plt.imshow(pooled_tensor.squeeze().numpy().transpose(1, 0), cmap='gray') plt.show() ,上述代码出现问题:segments = slic(x.numpy().transpose(1, 2, 0), n_segments=self.n_segments, compactness=10) ValueError: axes don't match array,如何修改
这个错误通常是由于输入的张量维度不匹配导致的。在这里,可能是由于输入张量的维度不是按照预期的顺序排列导致的。建议检查一下输入张量 x 的维度是否为 (batch_size, channels, height, width),如果不是,可以使用 PyTorch 的 permute 函数重新排列维度。您可以尝试将这一行代码修改为:
```
segments = slic(x.permute(0, 2, 3, 1).numpy(), n_segments=self.n_segments, compactness=10)
```
这样可以将维度从 (batch_size, channels, height, width) 转换为 (batch_size, height, width, channels),以适应 SLIC 函数的输入要求。
将这两个代码结合import cv2 import numpy as np import urllib.request import tensorflow as tf # 下载DeepLabv3+模型权重文件 model_url = "http://download.tensorflow.org/models/deeplabv3_mnv2_pascal_train_aug_2018_01_29.tar.gz" tar_filename = "deeplabv3_mnv2_pascal_train_aug.tar.gz" urllib.request.urlretrieve(model_url, tar_filename) # 解压缩 with tarfile.open(tar_filename, "r:gz") as tar: tar.extractall() model_filename = "deeplabv3_mnv2_pascal_train_aug/frozen_inference_graph.pb" # 加载模型 graph = tf.Graph() with graph.as_default(): od_graph_def = tf.GraphDef() with tf.io.gfile.GFile(model_filename, 'rb') as fid: serialized_graph = fid.read() od_graph_def.ParseFromString(serialized_graph) tf.import_graph_def(od_graph_def, name='') # 读取图像 image_path = "your_image.jpg" image = cv2.imread(image_path) # 进行图像分割 with tf.compat.v1.Session(graph=graph) as sess: input_tensor = graph.get_tensor_by_name('ImageTensor:0') output_tensor = graph.get_tensor_by_name('SemanticPredictions:0') output = sess.run(output_tensor, feed_dict={input_tensor: image}) # 解码并可视化分割结果 segmentation_mask = np.squeeze(output) segmentation_mask = np.uint8(segmentation_mask) segmentation_mask = cv2.resize(segmentation_mask, (image.shape[1], image.shape[0]), interpolation=cv2.INTER_NEAREST) # 显示原始图像和分割结果 cv2.imshow("Image", image) cv2.imshow("Segmentation Mask", segmentation_mask) cv2.waitKey(0) cv2.destroyAllWindows() model1 = models.CellposeModel(gpu=True, model_type='livecell') model2 = models.Cellpose(gpu=True,model_type='nuclei') model3= models.Cellpose(gpu=True,model_type='cyto2') 集成DeepLabv3+模型和cellpose模型
要将DeepLabv3+模型和Cellpose模型集成,你可以按照以下步骤进行修改:
```python
import cv2
import numpy as np
import urllib.request
import tensorflow as tf
from cellpose import models
# 下载DeepLabv3+模型权重文件
model_url = "http://download.tensorflow.org/models/deeplabv3_mnv2_pascal_train_aug_2018_01_29.tar.gz"
tar_filename = "deeplabv3_mnv2_pascal_train_aug.tar.gz"
urllib.request.urlretrieve(model_url, tar_filename)
# 解压缩
with tarfile.open(tar_filename, "r:gz") as tar:
tar.extractall()
model_filename = "deeplabv3_mnv2_pascal_train_aug/frozen_inference_graph.pb"
# 加载DeepLabv3+模型
graph = tf.Graph()
with graph.as_default():
od_graph_def = tf.GraphDef()
with tf.io.gfile.GFile(model_filename, 'rb') as fid:
serialized_graph = fid.read()
od_graph_def.ParseFromString(serialized_graph)
tf.import_graph_def(od_graph_def, name='')
# 加载Cellpose模型
model1 = models.CellposeModel(gpu=True, model_type='livecell')
model2 = models.CellposeModel(gpu=True, model_type='nuclei')
model3 = models.CellposeModel(gpu=True, model_type='cyto2')
# 读取图像
image_path = "your_image.jpg"
image = cv2.imread(image_path)
# 进行图像分割(DeepLabv3+模型)
with tf.compat.v1.Session(graph=graph) as sess:
input_tensor = graph.get_tensor_by_name('ImageTensor:0')
output_tensor = graph.get_tensor_by_name('SemanticPredictions:0')
output = sess.run(output_tensor, feed_dict={input_tensor: image})
# 解码并可视化分割结果(DeepLabv3+模型)
segmentation_mask = np.squeeze(output)
segmentation_mask = np.uint8(segmentation_mask)
segmentation_mask = cv2.resize(segmentation_mask, (image.shape[1], image.shape[0]), interpolation=cv2.INTER_NEAREST)
# 进行图像分割(Cellpose模型)
masks1, _, _, _ = model1.eval(image)
masks2, _, _, _ = model2.eval(image)
masks3, _, _, _ = model3.eval(image)
# 可视化结果
cv2.imshow("Original Image", image)
cv2.imshow("Segmentation Mask (DeepLabv3+)", segmentation_mask)
cv2.imshow("Cell Masks (Model 1)", masks1)
cv2.imshow("Cell Masks (Model 2)", masks2)
cv2.imshow("Cell Masks (Model 3)", masks3)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这样,你就可以同时使用DeepLabv3+模型和Cellpose模型进行图像分割和细胞分割了。请注意,你需要根据你的需求进行适当的调整和修改。
希望这可以帮助到你!如果还有其他问题,请随时告诉我。
相关推荐
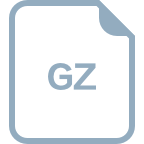











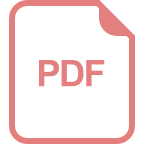