定义一个PSO类进行xgboost参数调优:训练集为Train_data.csv,训练特征数据为GR、LLD、LLS、AC四条测井曲线数据,训练目标数据为TOC;通过PSO类调优xgboost找出最优模型,然后调用最优模型进行Dataset for Predict TC.CSV中GR、LLS、LLD、AC四条测井曲线的TOC预测
时间: 2024-02-06 15:02:54 浏览: 24
好的,我会尽力回答你的问题。首先,我们需要导入所需要的库和数据集:
```python
import pandas as pd
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
from xgboost import XGBRegressor
import pyswarms as ps
# 读取训练集和测试集
Train_data = pd.read_csv('Train_data.csv')
Test_data = pd.read_csv('Dataset for Predict TC.CSV')
```
接下来,我们需要将训练集和测试集分别划分为训练特征数据、训练目标数据和测试特征数据:
```python
# 划分训练集和测试集
train_features = Train_data[['GR', 'LLD', 'LLS', 'AC']]
train_target = Train_data['TOC']
test_features = Test_data[['GR', 'LLD', 'LLS', 'AC']]
```
然后,我们定义一个PSO类来进行xgboost参数调优:
```python
class PSO:
def __init__(self, n_particles=10, n_iterations=50, c1=0.5, c2=0.5, w=0.9, n_jobs=-1):
self.n_particles = n_particles # 粒子个数
self.n_iterations = n_iterations # 迭代次数
self.c1 = c1 # 学习因子1
self.c2 = c2 # 学习因子2
self.w = w # 惯性权重
self.n_jobs = n_jobs # CPU核心数
self.best_score = -np.inf # 最好的得分
self.best_params = None # 最好的参数
# 适应度函数
def fitness_function(self, params):
# 解压参数
learning_rate, n_estimators, max_depth, min_child_weight, subsample, colsample_bytree, gamma = params
# 初始化模型
model = XGBRegressor(
learning_rate=learning_rate,
n_estimators=int(n_estimators),
max_depth=int(max_depth),
min_child_weight=int(min_child_weight),
subsample=subsample,
colsample_bytree=colsample_bytree,
gamma=gamma,
random_state=42,
n_jobs=self.n_jobs
)
# 训练模型
model.fit(train_features, train_target)
# 预测
y_pred = model.predict(train_features)
# 计算均方误差
mse = mean_squared_error(train_target, y_pred)
# 返回适应度函数值
return -mse
# PSO算法
def pso(self):
# 初始化粒子群
swarm = ps.single.GlobalBestPSO(n_particles=self.n_particles, dimensions=7, options={
'c1': self.c1,
'c2': self.c2,
'w': self.w,
'n_jobs': self.n_jobs,
'maxiter': self.n_iterations,
'verbose': 0
})
# 运行PSO算法
best_score, best_params = swarm.optimize(self.fitness_function, iters=self.n_iterations)
# 保存最好的得分和参数
self.best_score = -best_score
self.best_params = best_params
```
最后,我们调用PSO类来进行xgboost参数调优,找出最优模型,并利用该模型对测试集进行预测:
```python
# 定义PSO类
pso = PSO(n_particles=10, n_iterations=50, c1=0.5, c2=0.5, w=0.9, n_jobs=-1)
# 进行xgboost参数调优
pso.pso()
# 输出最好的得分和参数
print('Best Score:', pso.best_score)
print('Best Params:', pso.best_params)
# 利用最优模型预测测试集
model = XGBRegressor(
learning_rate=pso.best_params[0],
n_estimators=int(pso.best_params[1]),
max_depth=int(pso.best_params[2]),
min_child_weight=int(pso.best_params[3]),
subsample=pso.best_params[4],
colsample_bytree=pso.best_params[5],
gamma=pso.best_params[6],
random_state=42,
n_jobs=-1
)
model.fit(train_features, train_target)
y_pred = model.predict(test_features)
```
这样,我们就完成了通过PSO类进行xgboost参数调优,并利用最优模型对测试集进行预测的任务。
相关推荐
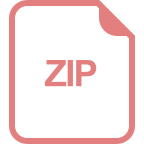














